Kanban views have long been a favorite for organizing and visualizing workflows in Odoo. These visually dynamic views provide an intuitive way to track project progress, manage tasks, and reorganize data on the fly. With Odoo 17, handle widgets elevate Kanban views to the next level by introducing drag-and-drop reordering and seamless record management.
This blog will guide you through the technical aspects of creating and managing handle widgets for Kanban views in Odoo 17 and the benefits to hire Odoo development company. By following these detailed steps, you can unlock the full potential of handle widgets and ensure your users enjoy a streamlined, user-friendly experience.
What Are Handle Widgets in Odoo 17?
Handle widgets in Odoo 17 are interactive UI components used in Kanban views to enable drag-and-drop functionality, allowing users to reorder records or move them between different groups (or stages). These widgets are particularly valuable in workflows where prioritization, sequencing, or categorization is required. Handle widgets provide a visual and intuitive way to manage tasks, projects, or any other record-based operations that demand dynamic organization.
# Key Characteristics of Handle Widgets
1. Drag-and-Drop Functionality:
- Handle widgets empower users to click and drag records to reorder them within a Kanban column or move them across columns (stages or groups).
- For example, in a task management module, users can drag a task from “To Do” to “In Progress” or reorder tasks within “To Do” based on priority.
2. Visual Indicators:
- Handle widgets are represented as icons (usually three horizontal lines resembling a “handle”) or areas on a record that signal where users can click to drag the record.
- These indicators enhance usability by making it visually clear where the drag-and-drop feature is enabled.
3. Dynamic Updates:
- When a user reorders records using handle widgets, the sequence field in the backend is updated automatically.
- The changes are often saved instantly without requiring a page refresh, ensuring real-time synchronization between the UI and database.
4. Back-End Integration:
- Handle widgets rely on a dedicated sequence field in the database model to determine the order of records.
- The sequence value updates when the user repositions a record, ensuring proper ordering for future operations or views.
# Why Are Handle Widgets Important in Odoo 17?
Handle widgets play a crucial role in improving usability and workflow management. Here’s why they are indispensable:
1. Enhanced User Experience:
- Users can interact with records intuitively without needing to edit fields or manually change sequence values.
- Drag-and-drop actions feel natural and reduce cognitive load.
2. Time-Saving:
- Tasks like prioritizing records, rearranging workflows, or managing pipelines are significantly faster with handle widgets compared to traditional manual updates.
3. Real-Time Record Management:
- Changes are instantly reflected in the database, ensuring that users and teams always work with the most up-to-date information.
4. Versatile Applications:
- Handle widgets can be used in various scenarios, including:
Task Management: Reorder tasks based on deadlines or priority.
Sales Pipelines: Move leads between stages, such as “Qualified,” “Negotiation,” and “Won.”
Project Workflows: Shift tasks between project milestones or teams.
Inventory Sorting: Adjust product listings based on preferences.
Benefits of Handle Widgets in Kanban View
- Drag-and-Drop Reordering: Users can reorder tasks or records effortlessly within and across stages.
- Improved Task Management: Ideal for task prioritization, pipeline workflows, or project management.
- Real-Time Updates: Changes made using handle widgets are reflected instantly in the database.
- Enhanced Visual Experience: Provides a clear and organized structure for managing records.
Step-by-Step Guide to Implement Handle Widgets in Odoo 17
Let’s break the process into manageable steps:
Step 1: Add a Sequence Field to the Model
The sequence field determines the order of the records in a Kanban view. If your model doesn’t already have a sequence field, you’ll need to add it.
Python Code: Adding Sequence Field
python
from odoo import models, fields class Task(models.Model): _name = 'project.task' _description = 'Task' _order = 'sequence' name = fields.Char(string="Task Name", required=True) sequence = fields.Integer(string="Sequence", default=10) stage_id = fields.Many2one('project.task.stage', string="Stage")
Explanation:
- The _order attribute specifies that records are sorted by sequence.
- The sequence field holds numeric values to control the order of tasks.
Step 2: Modify the Kanban View
Next, update the Kanban view in the XML file to include the handle widget.
XML Code for Kanban View with Handle Widget
xml
<record id="view_task_kanban" model="ir.ui.view"> <field name="name">project.task.kanban</field> <field name="model">project.task</field> <field name="arch" type="xml"> <kanban> <field name="sequence" widget="handle"/> <field name="stage_id"/> <templates> <t t-name="kanban-box"> <div t-att-class="'o_kanban_record'"> <div class="o_kanban_details"> <strong><field name="name"/></strong> </div> </div> </t> </templates> </kanban> </field> </record>
- Show the XML code for the Kanban view in your editor.
- Highlight the widget=”handle” part to emphasize the addition of the handle widget.
Step 3: Enable Grouping by Stages
To group tasks by stage, use the default_group_by attribute in the Kanban view.
Updated XML Code: Grouping by Stages
xml
<kanban default_group_by="stage_id"> <field name="sequence" widget="handle"/> <field name="stage_id"/> <templates> <t t-name="kanban-box"> <div t-att-class="'o_kanban_record'"> <div class="o_kanban_details"> <strong><field name="name"/></strong> </div> </div> </t> </templates> </kanban>
This groups tasks by their stage_id and allows drag-and-drop movement between stages.
- Show the Kanban view in Odoo with records grouped by stages.
Step 4: Test the Kanban View
- Restart the Odoo server and upgrade the module.
- Navigate to the module containing the Kanban view.
- Verify that:
- Show the Kanban view in action, with a record being dragged and dropped within the same stage.
– Records can be reordered within a stage using the handle widget.
– Records can be moved between stages by dragging and dropping.
Also Read: Custom kanban view
Advanced Configurations
1. Adding Color Indicators
Enhance the Kanban view by adding color-coded tags or indicators to represent priority levels, task status, or other attributes.
Example:
Adding Color Field
python
priority = fields.Selection([ ('0', 'Low'), ('1', 'Normal'), ('2', 'High'), ], default='1') color = fields.Integer(string="Color", compute="_compute_color") @api.depends('priority') def _compute_color(self): for task in self: task.color = {'0': 2, '1': 0, '2': 1}.get(task.priority, 0)
Update Kanban View with Color
xml
<div t-att-class="'o_kanban_record o_priority_color_' + record.color"> <strong><field name="name"/></strong> </div>
Display the updated Kanban view with tasks in different colors based on priority.
2. Restricting Drag-and-Drop Actions
In some scenarios, you may want to restrict drag-and-drop functionality to specific user roles or stages.
Python Code for Access Control
python
@api.model def create(self, vals): if not self.env.user.has_group('project.group_project_manager'): raise AccessError("You do not have the rights to reorder tasks.") return super(Task, self).create(vals)
- Show a warning message that appears when unauthorized users try to reorder records.
3. Adding Filters and Search Options
Add filters and search functionality to make it easier for users to find specific tasks.
XML Code: Filters in Kanban View
xml
<filter string="High Priority" domain="[('priority', '=', '2')]"/> <filter string="In Progress" domain="[('stage_id.name', '=', 'In Progress')]"/>
- The Kanban view with the filter dropdown visible.
Common Issues and Troubleshooting
1. Handle Widget Not Visible:
- Ensure the sequence field is present in the model.
- Verify the widget=”handle” attribute is correctly added.
2. Drag-and-Drop Not Working:
- Check browser console for JavaScript errors.
- Ensure proper permissions are assigned to the sequence field.
3. Sequence Not Updating:
- Ensure the _order attribute in the model is set to sequence.
Real-World Use Cases
1. Project Management
Use handle widgets to reorder tasks based on priority or deadlines, ensuring project timelines stay on track.
2. CRM Pipeline Management
Organize leads by dragging and dropping them between sales stages, improving team efficiency and visibility.
3. Manufacturing Workflows
Manage production workflows by moving records between different manufacturing stages, optimizing task assignments.
Bonus Read: Reasons to Hire an Odoo Agency in Canada
Why Choose Shiv Technolabs?
At Shiv Technolabs, we specialize in Odoo Kanban view customization and Odoo 17 handle widget implementation. Our expertise includes:
- Customizing Kanban views to fit your business needs.
- Implementing drag-and-drop functionality to enhance user workflows.
- Providing end-to-end Odoo customization services in Canada, including advanced widget integrations.
Contact Shiv Technolabs to create a powerful, intuitive Kanban system tailored to your requirements.
Conclusion
Handle widgets in Odoo 17 bring flexibility, ease of use, and enhanced functionality to Kanban views. By following this guide, you can implement drag-and-drop features, customize workflows, and create a seamless experience for your users.
If you’re looking for expert assistance with Odoo Kanban view configuration, Shiv Technolabs is here to help. Let us transform your business processes with innovative Odoo solutions.
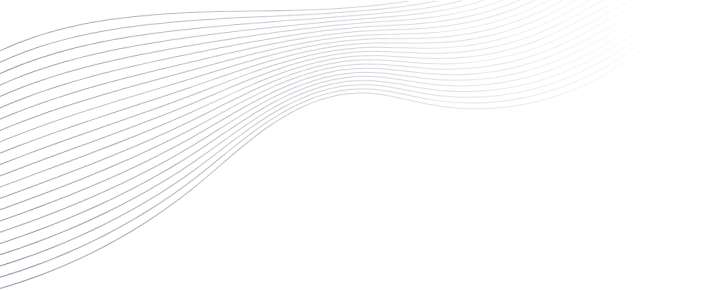
Revolutionize Your Digital Presence with Our Mobile & Web Development Service. Trusted Expertise, Innovation, and Success Guaranteed.