Blockchain technology has become a powerful solution across various industries, from finance to healthcare. The decentralized nature of blockchain, where data is stored across a network of nodes, provides resilience and transparency. However, the same features that make blockchain attractive also introduce vulnerabilities, making security a top priority in blockchain development.
Python has emerged as a favored language for blockchain developers due to its simplicity, readability, and the extensive range of libraries available for cryptography and blockchain functionality. In this blog, we’ll focus on building secure blockchain applications in Python and outline the critical security measures at each development stage to protect blockchain systems from vulnerabilities.
Getting Started with Blockchain Development in Python
Python offers a flexible and robust platform for developing blockchain applications. Here’s an overview of essential steps to begin blockchain development with Python.
# Key Python Libraries for Blockchain Development
Several libraries provide the necessary tools for blockchain development:
1. hashlib: A standard library for hash functions, which are essential for blockchain’s data integrity.
2. PyCryptodome: Offers a suite of cryptographic functions for encryption, decryption, and secure data storage.
3. Flask: Useful for creating APIs to interface with the blockchain.
4. Requests: Enables easy HTTP requests to interact with external blockchain APIs.
These libraries simplify the integration of blockchain’s core elements—hashing, encryption, and network communication.
# Setting Up the Development Environment
Before diving into code, set up an isolated environment using Python’s venv to prevent conflicts between libraries. Then, install necessary packages, particularly the ones mentioned above, to prepare your environment for blockchain coding.
# Building a Basic Blockchain in Python: An Introduction
Creating a basic blockchain in Python involves defining a Block and a Blockchain class. A block contains essential elements like index, timestamp, data, and the previous block’s hash. Each block in the chain points to its predecessor, creating an unbroken link.
A simple blockchain structure provides a foundation, but it lacks security features. As we progress, we’ll add cryptographic methods to secure the blockchain and implement consensus mechanisms for trust.
# Understanding Security Challenges in Blockchain Applications
Blockchain security encompasses protecting data integrity, preventing unauthorized access, and safeguarding against specific blockchain-related attacks. Knowing the threats is critical to mitigating them.
Common Security Threats in Blockchain Systems
- 51% Attack: When an entity gains control over more than 50% of the network’s mining power, it can manipulate transactions and alter the blockchain’s integrity.
- Sybil Attack: Involves creating multiple fake identities within the network to overwhelm legitimate users and disrupt the system’s functionality.
- Double-Spending: Occurs when an attacker spends the same cryptocurrency multiple times, exploiting a flaw in the verification process.
- Smart Contract Vulnerabilities: Weak or poorly coded smart contracts may contain exploitable logic, resulting in significant financial losses or data breaches.
# The Importance of Secure Coding in Python
To protect blockchain applications from these threats, developers must adopt secure coding practices and thoroughly test each component. Security should be embedded in the code structure rather than added as an afterthought, particularly for applications handling sensitive or financial data.
Implementing Cryptography for Blockchain Security
Cryptography is the backbone of blockchain security, enabling data protection and transaction integrity. Let’s discuss how Python makes it easier to integrate strong cryptographic methods into blockchain applications.
# Basics of Cryptography in Blockchain
In blockchain, cryptography secures data through two primary methods:
- Hash Functions: One-way functions like SHA-256 and SHA-3 transform data into a fixed-size string of characters. In a blockchain, each block’s hash contains the hash of the previous block, creating a “chain” that detects tampering.
- Asymmetric and Symmetric Encryption: Asymmetric encryption (using a public and private key pair) is fundamental to user authentication and transaction verification, while symmetric encryption can protect sensitive data during transmission.
# Using Python Libraries for Cryptography
Python’s hashlib provides hash functions that are straightforward to use. For instance, hashing a block’s data ensures it hasn’t been altered, a primary step in preventing data tampering. The PyCryptodome library allows developers to implement advanced cryptographic protocols like AES (Advanced Encryption Standard), ensuring data remains confidential throughout the process.
# Securely Storing and Transmitting Blockchain Data
Encrypting data before storing it within a block offers an added layer of security. Data stored on the blockchain is accessible to every participant, making encryption critical for sensitive information. With PyCryptodome, data can be encrypted with minimal code while ensuring strong security standards.
Best Practices for Writing Secure Blockchain Code in Python
Writing secure code is paramount in blockchain development. This section outlines best practices to protect your Python-based blockchain application from common vulnerabilities.
# Ensuring Data Integrity and Authenticity
Data integrity in blockchain is achieved through secure hashing and validation. Each block must be verified by comparing its hash with the previous block’s hash. In Python, regularly hashing the data with hashlib and storing the results ensures that any tampering can be detected immediately. Use multi-layered validation processes for user actions and transaction data to keep data integrity intact.
# Managing User Authentication and Permissions
Strong authentication mechanisms are essential for controlling access in blockchain applications. By implementing multi-factor authentication and unique cryptographic keys for each user, developers can safeguard user accounts. Python libraries like PyCryptodome support these functions, allowing developers to manage permissions and user roles efficiently.
# Using Smart Contracts: Avoiding Common Vulnerabilities
Smart contracts automate transactions within a blockchain. However, poorly coded smart contracts are highly vulnerable. To write secure smart contracts, adopt coding practices that avoid reentrancy attacks, overflows, and race conditions. Python’s simplicity can help in structuring clean, readable smart contracts and minimizing complex, vulnerable logic.
# Securing the Python Codebase: Linters, Code Review, and Testing
Maintaining code quality and security standards is crucial in blockchain development. Employ linters, such as pylint, to catch potential bugs and enforce coding standards. Additionally, conduct regular code reviews with peers and use automated testing to identify and rectify vulnerabilities. In Python, libraries like pytest facilitate extensive testing, which can uncover vulnerabilities before they reach production.
# Implementing Secure Consensus Mechanisms
Consensus protocols are the backbone of decentralized networks, ensuring agreement among all participants. Choosing the right mechanism is key to securing a blockchain network.
Overview of Consensus Protocols: Proof of Work, Proof of Stake, and Delegated Proof of Stake
Different consensus protocols offer varying levels of security:
- Proof of Work (PoW): The original consensus protocol, PoW relies on solving complex mathematical puzzles. It’s secure but resource-intensive.
- Proof of Stake (PoS): Validators are selected based on the amount of cryptocurrency they hold, making it more energy-efficient than PoW.
- Delegated Proof of Stake (DPoS): A more democratic approach, DPoS allows users to vote for validators, balancing efficiency with decentralization.
# Implementing Proof of Work in Python
To add Proof of Work (PoW) to a Python blockchain, developers can create a simple “mining” function that adjusts the difficulty level by requiring a specific hash format. This not only adds security but also prevents spam transactions. Python’s hashlib is ideal for this, enabling hash-based validation.
# Pros and Cons of Various Consensus Mechanisms in Terms of Security
PoW is secure but requires extensive resources. PoS reduces the environmental impact but may introduce centralization risks if large stakeholders dominate. DPoS offers a more democratic model, but its security depends on the network’s voting behavior. Select a consensus mechanism based on the specific security and resource needs of your application.
Data Privacy in Blockchain Applications
Data privacy is crucial, especially in public blockchains where information is accessible to all participants. Here’s how to address privacy concerns in blockchain applications.
# Addressing Privacy Concerns in Public Blockchains
Since blockchain data is typically open, sensitive information must be protected. By anonymizing data, developers can safeguard privacy without compromising the transparency of the blockchain. Python libraries like PyCryptodome allow developers to implement advanced privacy measures, such as pseudonymization or encryption.
# Anonymizing Data with Python-Based Tools
Python offers tools like cryptography for masking user identities. Implementing pseudonyms instead of actual identities and encrypting sensitive data ensures user privacy while keeping transactions verifiable. For high-security needs, consider integrating homomorphic encryption, which allows computations on encrypted data.
# Implementing Zero-Knowledge Proofs (ZKPs) in Python for Enhanced Privacy
Zero-Knowledge Proofs (ZKPs) are a powerful method to verify information without revealing the actual data. ZKPs can verify user information while maintaining anonymity. Python can support ZKPs through custom implementations or external libraries, allowing for a flexible privacy solution in sensitive blockchain applications.
Testing and Validating Security in Blockchain Applications
Testing is essential to ensure the security of blockchain applications. This involves using various testing approaches and tools to uncover any vulnerabilities.
# Importance of Unit Testing and Test-Driven Development (TDD)
Unit testing each part of the blockchain application can catch issues early. Test-Driven Development (TDD), where tests are written before the actual code, can enforce security practices. Python’s unittest and pytest frameworks facilitate these testing methods, allowing developers to write efficient tests for each component.
# Testing Blockchain Security: Penetration Testing and Vulnerability Scanning
Penetration testing simulates attacks to evaluate the application’s security defenses. In Python, tools like scapy and sqlmap help simulate attacks and identify weaknesses in code, networks, and APIs. Regular vulnerability scans should be part of your testing process to ensure continued protection against emerging threats.
# Python Testing Libraries and Tools (e.g., unittest, pytest, hypothesis)
Python’s hypothesis library is useful for property-based testing, where random inputs are generated to test how the blockchain handles unusual cases. Combining unittest, pytest, and hypothesis provides a robust testing suite that ensures the security of the application under various conditions.
Deploying Blockchain Applications Securely
The deployment phase is where your blockchain application goes live, requiring secure handling to maintain its integrity and protect it from external threats.
# Securing the Deployment Environment: Servers, APIs, and Nodes
Choose a secure hosting solution for the blockchain’s servers, whether it’s on-premises or cloud-based. Secure APIs to prevent unauthorized access, and encrypt node communication to keep data secure. Restrict access using firewalls, secure shells, and encryption protocols.
# Steps for Securely Deploying Blockchain Applications on Cloud
If deploying on the cloud, implement strict access controls and configure your cloud environment for data security. Use Python’s compatibility with cloud platforms (like AWS or Azure) to integrate automated security checks that monitor application health and activity logs.
# Monitoring and Updating Blockchain Applications Post-Deployment
Regular updates are critical for maintaining blockchain security. After deployment, implement automated monitoring tools to detect anomalies, monitor API usage, and alert on suspicious activity. Keep libraries and dependencies up-to-date to protect against new vulnerabilities.
Conclusion
In today’s fast-evolving digital landscape, building secure blockchain applications is vital for any developer working in this field. By leveraging Python’s extensive toolkit and following best practices in security, cryptography, testing, and deployment, developers can create blockchain applications that are both innovative and resilient. The future of blockchain depends on secure, transparent, and privacy-conscious development—achieving this requires a commitment to continuous learning and rigorous application of security standards.
For those looking to build secure and reliable blockchain applications, Shiv Technolabs is here to support your vision with a team of skilled developers dedicated to delivering outstanding results. As a leading Python development company, we specialize in providing robust Python app development services in Turkey that meet industry standards. Whether you’re at the concept stage or ready for deployment, our experts can guide you through every step. When you choose to hire Python developers from Shiv Technolabs, you gain a team committed to ensuring the security, scalability, and efficiency of your blockchain solutions. Let us bring your next-gen application to life—securely and successfully.
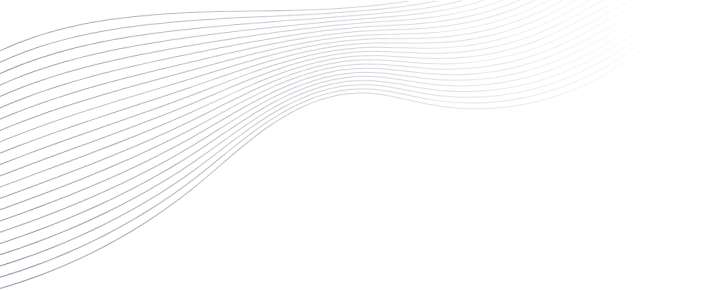
Revolutionize Your Digital Presence with Our Mobile & Web Development Service. Trusted Expertise, Innovation, and Success Guaranteed.