Table of Contents
Laravel is a very flexible and extensible PHP framework well known for building modern applications. Very few features it has are more powerful than having the ability to create custom packages that extend its functionalities for applications. Custom Laravel packages development process can encapsulate your code for reuse in various projects, but for a package to be really effective, it is necessary to understand how to work with important aspects like Service Providers, Facades, and Config files.
Let’s see more about developing a Custom Laravel service provider and facade implementation by using these important components in a very simple and understandable manner.
What is a Laravel Package?
A Laravel package is an acquisition of reusable code that can be shared across different Laravel applications. It can be as simple as a small utility or as complex as an entire feature for a project. For instance, you might develop a package to handle image uploads, payment processing, or user authentication. Laravel’s package development system provides a powerful structure to make sure that these packages are modular, maintainable, and easy to integrate.
A typical Laravel package might involve routes, controllers, views, models, migrations, and other features. You need to register your package with Laravel to integrate such functionality into your app, which is where Service Providers, Facades, and Config files come into play.
# Service Providers
Service Providers are in charge of bootstrapping the components of your application in Laravel. They act as the main place where all of your application’s services are configured and registered. The Service Provider is responsible for registering your package’s functionality while developing a package, binding services to the container, and booting any necessary actions.
Why Service Providers Matter?
Service Providers are crucial in Laravel packages as they allow you to integrate your package’s features seamlessly into the Laravel service container. Your package would not be able to interact with Laravel’s core features without them which includes dependency injection, events, and routing.
Creating a Service Provider
Follow these steps to create a Service Provider for your package:
1. Create a Service Provider Class
Create a src folder (if it doesn’t exist already) in your package’s directory. Inside src, create a directory called Providers. Then, within this directory, create a new file, PackageServiceProvider.php.
<?php namespace YourPackage\Providers; use Illuminate\Support\ServiceProvider; class PackageServiceProvider extends ServiceProvider { public function register() { // Register package services } public function boot() { // Perform package-specific bootstrapping } }
2. Register Your Service Provider
In order for Laravel to know about your Service Provider, you must register it in the config/app.php file of the consuming application (or within your package’s composer.json file, if you want this to be automatic).
'providers' => [ // Other service providers... YourPackage\Providers\PackageServiceProvider::class, ],
3. Register Package Services in the register() Method
This method is to help you bind classes or services into the Laravel service container. For instance, if you want to bind a service class to the container, you can do so here.
public function register() { $this->app->singleton('YourPackageService', function ($app) { return new YourPackageService(); }); }
4. Bootstrapping in the boot() Method
The boot() method is where you can perform any additional setup or configuration after the services have been registered. This could include setting up routes, views, or event listeners.
public function boot() { // Load routes from a routes file $this->loadRoutesFrom(__DIR__.'/../routes/web.php'); // Load views $this->loadViewsFrom(__DIR__.'/../resources/views', 'yourpackage'); }
Registering Routes, Views, and Migrations
You can use the boot() method to register routes, views, and migrations:
public function boot() { // Register Routes $this->loadRoutesFrom(__DIR__.'/../routes/web.php'); // Register Views $this->loadViewsFrom(__DIR__.'/../resources/views', 'yourpackage'); // Register Migrations $this->loadMigrationsFrom(__DIR__.'/../database/migrations'); }
# Facades: Simplifying Access to Your Package
Facades provide a simple interface to access services in the Laravel container. They allow you to call methods on services without needing to inject the dependencies manually. Using facades in your package helps to provide a clean and easy-to-use syntax for consumers of your package.
Why Facades Matter?
Facades make accessing services from the service container straightforward and intuitive in Laravel. Creating a facade allows users of your package to easily interact with the services your package provides while building a Laravel package.
Creating a Facade
Follow these steps to create a facade for your package:
- Create a Facade Class
Inside your package, create a new Facade class. It should extend Illuminate\Support\Facades\Facade and implement the getFacadeAccessor() method, which returns the service container key.
<?php namespace YourPackage\Facades; use Illuminate\Support\Facades\Facade; class YourPackageFacade extends Facade { protected static function getFacadeAccessor() { return 'YourPackageService'; //The service registered in the Service Provider } }
- Alias the Facade in config/app.php
In the consuming Laravel application, you need to add an alias for the facade in the config/app.php file:
'aliases' => [ // Other aliases... 'YourPackage' => YourPackage\Facades\YourPackageFacade::class, ],
- Using the Facade
Now, you can easily use the facade in the consuming application:
use YourPackage; YourPackage::doSomething();
# Config Files: Making Your Package Customizable
Config files allow users of your package to customize its behavior. A config file can interpret settings such as API keys, database connections, or other options that users might want to configure.
Why Config Files Matter?
Config files provide a way to make your package flexible and customizable. Instead of hardcoding values into your package, you can provide a configuration file where users can easily update values according to their needs.
Creating Config Files
- Publish the Config File
You can include a default configuration file in your package, and then allow users to publish it to their application’s config directory.
First, create a config directory inside your package and add a yourpackage.php configuration file:
<?php return [ 'api_key' => env('YOURPACKAGE_API_KEY', 'default-api-key'), ];
- Publishing the Config File
In your PackageServiceProvider, use the publishes() method to allow the user to publish the config file.
public function boot() { $this->publishes([ __DIR__.'/../config/yourpackage.php' => config_path('yourpackage.php'), ]); }
- Accessing the Config Values
To access the configuration values within your package, you can use Laravel’s config() helper function:
$apiKey = config('yourpackage.api_key');
Conclusion
Developing Laravel custom package development services using Service Providers, Facades, and Config files offers a powerful way to extend the functionality of your applications. You can create flexible, reusable packages that integrate seamlessly with Laravel’s core features by understanding how to use these components. Service Providers enable you to register and bootstrap your package’s services, while Facades offer a simple interface for easy access to those services. Config files make your package customizable, allowing users to tailor it to their needs.
At Shiv Technolabs, we hire Laravel developers to help you in building custom Laravel solutions that leverage these principles, helping businesses create scalable, maintainable, and efficient web applications with ease as it is a Laravel package development company.
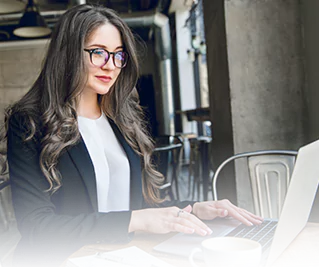