Table of Contents
React is the most popular JavaScript library which has made developing rich and complex user interfaces just a cakewalk for developers. You can also order custom ReactJS components with TypeScript. However when React is coupled with TypeScript- a statically typed superset of JavaScript-it comes with the additional benefit of in fact fewer runtime errors, better code maintainability, plus rapport-building among developers.
It may be seen that newbies might find it really tricky to deal with a manifold of states and the React Context API along with Typescript. This article will navigate through the ways to use TypeScript with React in a way to handle complicated states in React, especially focusing on the Context API.
Why Use TypeScript with React?
Before diving into state management, let’s quickly review why TypeScript is such a great match with React.
1. Type Safety
TypeScript helps catch errors during development, which would otherwise be found only at runtime in JavaScript. This reduces the chances of bugs and makes refactoring code much easier.
2. Better Developer Experience
You receive functionalities such as autocompletion, inline documentation, and type checking in your editor with TypeScript, thus making the development process all the faster and less error-prone.
3. Readability and Maintainability
Type annotations help other developers understand the structure of your components and data, which is especially useful when working on large projects with many contributors.
Now, let’s explore how TypeScript fits into handling complex state and the Context API in React.
Handling Complex State in React with TypeScript
State management is the most important aspect of building some dynamic UIs with React. Simple states, like a boolean or a string, are pretty straightforward; but handling very complex states such as objects, arrays, or any kind of deeply nested data can be quite tricky especially when it needs to be passed down many components.
With TypeScript, you can clearly declare the types for your state, making quite an easy task handling complex states.
Let’s look at an example where we manage a complex state object.
# Example: Managing a List of Users
Imagine we are building an app that displays a list of users. The state of the app might look like this:
interface User { id: number; name: string; email: string; } interface AppState { users: User[]; loading: boolean; error: string | null; } const App = () => { const [state, setState] = useState<AppState>({ users: [], loading: false, error: null, }); const fetchUsers = async () => { setState({ ...state, loading: true }); try { const response = await fetch('/api/users'); const data = await response.json(); setState({ users: data, loading: false, error: null }); } catch (err) { setState({ users: [], loading: false, error: 'Failed to fetch users' }); } }; return ( <div> <button onClick={fetchUsers}>Fetch Users</button> {state.loading ? <p>Loading...</p> : null} {state.error && <p>Error: {state.error}</p>} <ul class="orangeList"> {state.users.map(user => ( <li key={user.id}>{user.name}</li> ))} </ul> </div> ); };
Key Points:
- The AppState interface defines the shape of the state object. This makes sure that users, loading, and error are always present in the state and are of the correct types.
- By using TypeScript, the state structure is clear, which helps prevent errors like trying to access a property that doesn’t exist.
Using React Context API with TypeScript
Indeed, React comes up with the Context API so that any state can be shared throughout the component tree. It is not necessary to manually pass props along every level. This way is particularly great when there might be complex states, as it would not need a prop-drilling but offers a cleaner and more manageable code.
Let’s see how to combine TypeScript with the Context API to handle a complex state.
# Example: Managing Authentication State
We can build a complete authentication system through Context API and typescript. Authentication state will be stored in contexts like login status and profile details.
1. Create Context Types
First, we define the types for our context state and actions.
interface User { id: number; name: string; } interface AuthContextType { user: User | null; isAuthenticated: boolean; login: (user: User) => void; logout: () => void; }
2. Create the Context
Next, we specify the default value by creating the context itself using React.createContext.
import React, { createContext, useState, ReactNode } from 'react'; const defaultAuthContext: AuthContextType = { user: null, isAuthenticated: false, login: () => {}, logout: () => {}, }; const AuthContext = createContext<AuthContextType>(defaultAuthContext);
3. Create the Provider Component
Now we create the provider that will wrap our application and provide the context to all components that need access to it.
interface AuthProviderProps { children: ReactNode; } const AuthProvider: React.FC<AuthProviderProps> = ({ children }) => { const [user, setUser] = useState<User | null>(null); const login = (user: User) => { setUser(user); }; const logout = () => { setUser(null); }; return ( <AuthContext.Provider value={{ user, isAuthenticated: !!user, login, logout }}> {children} </AuthContext.Provider> ); };
4. Consuming the Context
Finally, any component that needs to consume the authentication state can use the useContext hook. Here’s how we can access the context in a component:
import React, { useContext } from 'react'; const UserProfile: React.FC = () => { const { user, isAuthenticated, logout } = useContext(AuthContext); if (!isAuthenticated) { return <p>Please log in</p>; } return ( <div> <h2>Welcome, {user?.name}!</h2> <button onClick={logout}>Log Out</button> </div> ); };
Key Points:
- By defining the AuthContextType, TypeScript ensures that all consuming components have access to the proper properties and methods.
- The AuthProvider component will handle the authentication state and expose the login and logout methods to the rest of the application.
- Using useContext, we reach the authentication state and provide the user with a personalized experience.
Conclusion
Handling complex state with Context API in React can be quite a hassle, but with TypeScript, it’s a lot more manageable. With clear types defined for your state and actions, TypeScript helps you avoid bugs and makes your codebase much more maintainable. You can share state across your components in a safe and predictable way using React’s Context API in combination with TypeScript, making it easier to build scalable, large, complex applications.
Therefore, with such functionality as in a simple app to a huge enterprise, usage of TypeScript and the React Context API optimization services becomes powerful instrumentation to address the challenge of complex state management in general.
Shiv Technolabs, a ReactJS TypeScript development company for scalable apps, helps businesses create robust, error-free, and efficient applications as we hire ReactJS developers with TypeScript expertise. We ensure smooth development, better performance, and seamless user experiences with these experts.
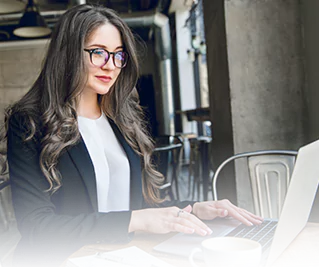