Table of Contents
Integrating a local payment gateway into a Django application is crucial for enabling smooth transactions in e-commerce platforms, especially if you are operating in a specific region like the UAE. This guide will walk you through the steps to integrate popular UAE payment gateways such as Payfort or Telr into your Django application.
1) Setting Up Your Django Environment
Before integrating a payment gateway, you need to ensure that your Django environment is properly set up. Start by creating a new Django project and app if you haven’t already.
django-admin startproject myproject cd myproject django-admin startapp payments
Add your new app to the INSTALLED_APPS list in settings.py:
# myproject/settings.py INSTALLED_APPS = [ ... 'payments', ... ]
2) Installing Required Packages
You’ll need the requests library to handle HTTP requests to the payment gateway’s API. Install it using pip:
pip install requests
3) Configuring the Payment Gateway
# Payfort
- Create an Account: First, create an account with Payfort and obtain your merchant identifier, access code, and SHA request phrase.
- Settings: Store these credentials in your Django settings file:
# myproject/settings.py PAYFORT_MERCHANT_IDENTIFIER = 'your_merchant_identifier' PAYFORT_ACCESS_CODE = 'your_access_code' PAYFORT_SHA_REQUEST_PHRASE = 'your_sha_request_phrase' PAYFORT_SANDBOX_URL = 'https://sbpaymentservices.payfort.com/FortAPI/paymentApi' PAYFORT_PRODUCTION_URL = 'https://paymentservices.payfort.com/FortAPI/paymentApi'
# Telr
- Create an Account: Similarly, create an account with Telr and get your store ID and auth key.
- Settings: Store these credentials in your settings:
# myproject/settings.py TELR_STORE_ID = 'your_store_id' TELR_AUTH_KEY = 'your_auth_key' TELR_SANDBOX_URL = 'https://secure.telr.com/gateway/order.json' TELR_PRODUCTION_URL = 'https://secure.telr.com/gateway/order.json'
4) Creating Payment Models
Create models to handle payment transactions. Here is a simple example:
# payments/models.py from django.db import models class Payment(models.Model): STATUS_CHOICES = ( ('initiated', 'Initiated'), ('completed', 'Completed'), ('failed', 'Failed'), ) amount = models.DecimalField(max_digits=10, decimal_places=2) status = models.CharField(max_length=20, choices=STATUS_CHOICES, default='initiated') transaction_id = models.CharField(max_length=100, unique=True, null=True, blank=True) created_at = models.DateTimeField(auto_now_add=True) def __str__(self): return f"Payment {self.id} - {self.status}"
Run the migrations to create the table:
python manage.py makemigrations python manage.py migrate
5) Creating Payment Views
Create views to handle payment initiation and response handling. For example, let’s create views for Payfort.
# payments/views.py import hashlib import json import requests from django.conf import settings from django.shortcuts import render, redirect from .models import Payment def generate_signature(params, sha_phrase): sorted_params = sorted(params.items()) concatenated_params = ''.join(f"{key}={value}" for key, value in sorted_params) concatenated_params = sha_phrase + concatenated_params + sha_phrase return hashlib.sha256(concatenated_params.encode('utf-8')).hexdigest() def initiate_payfort_payment(request): if request.method == 'POST': amount = request.POST.get('amount') payment = Payment.objects.create(amount=amount) params = { 'merchant_identifier': settings.PAYFORT_MERCHANT_IDENTIFIER, 'access_code': settings.PAYFORT_ACCESS_CODE, 'merchant_reference': payment.id, 'amount': int(float(amount) * 100), 'currency': 'AED', 'language': 'en', 'command': 'AUTHORIZATION', 'customer_email': 'customer@example.com', 'return_url': request.build_absolute_uri('/payments/payfort_callback/'), } signature = generate_signature(params, settings.PAYFORT_SHA_REQUEST_PHRASE) params['signature'] = signature response = requests.post(settings.PAYFORT_SANDBOX_URL, data=json.dumps(params), headers={'Content-Type': 'application/json'}) response_data = response.json() if response_data['status'] == 20: # success status code payment.transaction_id = response_data['fort_id'] payment.status = 'completed' payment.save() return redirect('payment_success') else: payment.status = 'failed' payment.save() return redirect('payment_failure') return render(request, 'payments/initiate_payfort_payment.html') def payfort_callback(request): # Handle Payfort callback response Pass
Also Read:- Can We Use Python as a Backend for a Flutter App?
6) Creating Templates
Create templates to handle the payment initiation form and success/failure pages.
<!-- templates/payments/initiate_payfort_payment.html --> <form method="post"> {% csrf_token %} <label for="amount">Amount:</label> <input type="text" name="amount" required> <button type="submit">Pay with Payfort</button> </form> ===================== ===================== <!-- templates/payments/payment_success.html --> <h1>Payment Successful!</h1> <p>Thank you for your payment.</p> ===================== ===================== <!-- templates/payments/payment_failure.html --> <h1>Payment Failed</h1> <p>Sorry, your payment could not be processed. Please try again.</p>
7) Updating URLs
Add the new views to your URL configuration.
# payments/urls.py from django.urls import path from .views import initiate_payfort_payment, payfort_callback urlpatterns = [ path('payfort/', initiate_payfort_payment, name='initiate_payfort_payment'), path('payfort_callback/', payfort_callback, name='payfort_callback'), ]
Include these URLs in the main URL configuration.
# myproject/urls.py from django.contrib import admin from django.urls import include, path urlpatterns = [ path('admin/', admin.site.urls), path('payments/', include('payments.urls')), ]
8) Testing the Integration
Run your Django server and test the payment integration:
python manage.py runserver
Visit the payment initiation URL (e.g., http://localhost:8000/payments/payfort/) and follow the payment process. Check your database to ensure the payment status updates correctly.
9) Handling Payment Responses
Ensure your callback view handles the response from the payment gateway correctly. This will typically involve verifying the payment status and updating your payment records.
# payments/views.py def payfort_callback(request): response_data = request.GET # or request.POST, depending on the gateway's response method merchant_reference = response_data.get('merchant_reference') status = response_data.get('status') fort_id = response_data.get('fort_id') payment = Payment.objects.get(id=merchant_reference) if status == '14': # Payfort success status code payment.status = 'completed' payment.transaction_id = fort_id else: payment.status = 'failed' payment.save() return redirect('payment_success' if payment.status == 'completed' else 'payment_failure')
Also Read:- Logging with Python and Loguru: A Step-by-Step Guide
Shiv Technolabs Can Help Simplify Your Django Payment Gateway Integration
Integrating a payment gateway can be a complex process, especially when dealing with multiple providers and ensuring compliance with local regulations. Shiv Technolabs, a leading software development company, can streamline this process for you. Our team of experienced developers specializes in Django and has extensive experience integrating various local and international payment gateways, including Payfort and Telr, into robust and secure e-commerce platforms.
# Expertise in Local Payment Solutions
At Shiv Technolabs, we understand the unique requirements of businesses operating in the UAE. We are well-versed in the specific functionalities and compliance standards required by local payment gateways. This ensures that your payment integration is not only functional but also adheres to all necessary legal and financial regulations. Our team stays updated with the latest developments and updates from payment gateway providers to offer you the most efficient and secure integration solutions.
# Comprehensive Integration Services
Our services encompass the full spectrum of payment gateway integration. This includes:
- Consultation and Planning: We start by understanding your business needs and objectives to recommend the best payment gateway for your requirements. We provide a detailed plan outlining the integration process and timeline.
- Development and Integration: Our developers handle the complete technical integration of the payment gateway with your Django application. This includes setting up secure transaction processes, handling callbacks, and ensuring data encryption.
- Testing and Quality Assurance: We conduct rigorous testing to ensure that the payment gateway integration is seamless and performs well under various scenarios. This includes testing for transaction success, failure handling, and edge cases.
- Ongoing Support and Maintenance: Post-integration, we offer continuous support and maintenance services. This ensures that your payment system remains operational and secure as your business grows and as payment gateways evolve.
# Why Choose Shiv Technolabs?
Choosing Shiv Technolabs means partnering with a company that values quality, security, and customer satisfaction. Our proven track record in delivering successful payment gateway integrations for businesses in UAE speaks volumes about our expertise and commitment. We aim to provide solutions that not only meet your current needs but also scale with your business.
By leveraging our services, you can focus on what you do best—growing your business—while we handle the technical complexities of payment gateway integration. Contact Shiv Technolabs today to discuss your project and find out how we, as a leading Python development company in UAE, can assist you in integrating local payment gateways with Django.
Conclusion
Integrating local payment gateways like Payfort or Telr into your Django application involves several steps, including setting up your environment, configuring the gateway, creating payment models, views, and handling payment responses. By following this guide, you can enable secure and efficient transactions in your Django projects tailored for the UAE market.
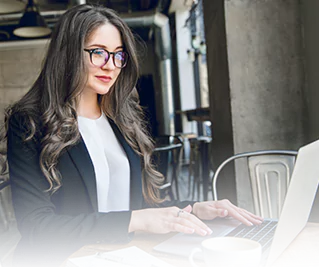