Table of Contents
In modern software development, databases play a crucial role in handling and managing data efficiently. Oracle Database is a leading choice for enterprises due to its robustness, scalability, and security features. Meanwhile, Python has become one of the most preferred programming languages for developers due to its simplicity, flexibility, and rich ecosystem.
When businesses need to interact with Oracle databases using Python, establishing a secure and efficient connection is key. Whether you’re fetching data, running queries, or integrating Oracle with Python-based applications, understanding the right approach ensures smooth performance.
This guide covers everything you need to connect Python with Oracle, from installing necessary packages to executing SQL queries and handling best practices.
Why Connect Python with Oracle?
Oracle databases store vast amounts of critical business data, and Python serves as a powerful tool for analyzing, processing, and managing that data efficiently. Here’s why integrating Python with Oracle Database is beneficial:
- Automated Data Processing: Python scripts can handle bulk data operations, making it easier to process and analyze information.
- Data Extraction for Analytics: Fetching data from Oracle databases into Python enables businesses to perform advanced analytics, data visualization, and machine learning tasks.
- Enterprise Applications: Python-based applications can directly communicate with Oracle databases for CRUD (Create, Read, Update, Delete) operations.
- Performance Optimization: Using Python, developers can optimize database queries, batch processes, and automate database tasks effectively.
With these advantages in mind, let’s move on to how to connect Python with Oracle database seamlessly.
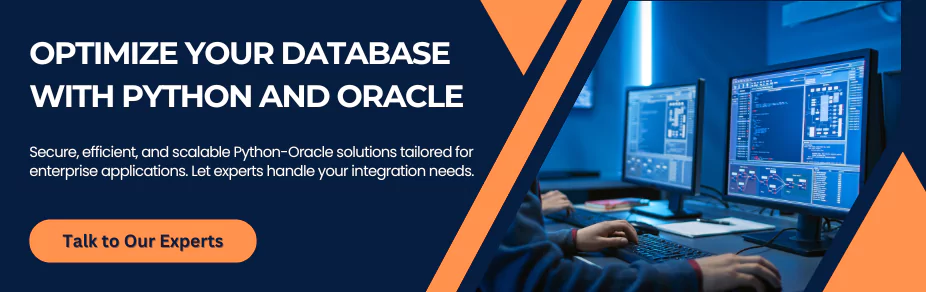
Step 1: Install Required Packages
To connect Oracle with Python, you need the cx_Oracle package, which is an efficient interface for Oracle databases.
1. Install cx_Oracle in Python
To install cx_Oracle, run the following command:
bash
pip install cx_Oracle
This package provides a Python interface to Oracle databases and is the primary library used for database operations.
2. Install Oracle Instant Client
Since cx_Oracle requires Oracle Client Libraries, you need to install Oracle Instant Client. Follow these steps:
- Download the latest Oracle Instant Client from Oracle’s official website.
- Extract the downloaded file into a directory (e.g., C:\oracle\instantclient_19_8 on Windows).
- Set the environment variable for Oracle Client:
- On Windows: Add C:\oracle\instantclient_19_8 to the PATH variable.
- On Linux/macOS: Add the directory path to the LD_LIBRARY_PATH or DYLD_LIBRARY_PATH.
Once these installations are complete, you are ready to establish a connection.
Step 2: Establish a Connection Between Python and Oracle
To successfully connect Python with Oracle, you need connection credentials like:
- Host
- Port (default: 1521)
- Service Name or SID
- Username
- Password
Here’s a Python script to establish a connection:
python
import cx_Oracle # Connection parameters dsn_tns = cx_Oracle.makedsn("hostname", 1521, service_name="your_service_name") conn = cx_Oracle.connect(user="your_username", password="your_password", dsn=dsn_tns) print("Connected to Oracle Database successfully!") # Close the connection conn.close()
Explanation:
- cx_Oracle.makedsn() constructs a connection string using hostname, port, and service name.
- cx_Oracle.connect() establishes the database connection using provided credentials.
- Finally, conn.close() ensures the connection is closed after use.
If using Oracle SID instead of service name, modify dsn_tns:
python
dsn_tns = cx_Oracle.makedsn("hostname", 1521, sid="your_sid")
Step 3: Executing SQL Queries in Python
Once connected, you can execute SQL queries to interact with Oracle databases.
1. Fetch Data from Oracle Table
To retrieve data from an Oracle table, use the following script:
python
import cx_Oracle dsn_tns = cx_Oracle.makedsn("hostname", 1521, service_name="your_service_name") conn = cx_Oracle.connect(user="your_username", password="your_password", dsn=dsn_tns) cursor = conn.cursor() # SQL Query query = "SELECT * FROM employees WHERE department = 'IT'" cursor.execute(query) # Fetch results for row in cursor.fetchall(): print(row) cursor.close() conn.close()
This script:
- Connects to Oracle.
- Executes an SQL query to fetch data from the employees table.
- Iterates over the results and prints them.
2. Insert Data into Oracle Table
To insert records into an Oracle table:
python
query = """INSERT INTO employees (id, name, department, salary) VALUES (:1, :2, :3, :4)""" data = (101, "John Doe", "IT", 70000) cursor.execute(query, data) conn.commit() print("Data inserted successfully!")
Using conn.commit() ensures the data is saved in the database.
3. Update Data in Oracle Table
To update existing records:
python
query = "UPDATE employees SET salary = :1 WHERE id = :2" cursor.execute(query, (75000, 101)) conn.commit() print("Record updated successfully!")
4. Delete Data from Oracle Table
To delete a record:
python
query = "DELETE FROM employees WHERE id = :1" cursor.execute(query, (101,)) conn.commit() print("Record deleted successfully!")
This approach helps in keeping the database clean and optimized.
Step 4: Handling Best Practices for Python-Oracle Integration
When working with databases, best practices ensure performance optimization and secure handling of sensitive data.
# Use Connection Pooling
Instead of opening and closing database connections frequently, use connection pooling to improve efficiency.
python
pool = cx_Oracle.SessionPool("username", "password", "hostname:1521/service_name", min=2, max=5, increment=1) conn = pool.acquire() cursor = conn.cursor()
# Use Bind Variables
Using bind variables in SQL queries enhances security and performance:
python
query = "SELECT * FROM employees WHERE department = :dept" cursor.execute(query, dept="HR")
# Implement Exception Handling
Always wrap database operations in try-except blocks to handle errors gracefully.
python
try: conn = cx_Oracle.connect("username", "password", "hostname:1521/service_name") cursor = conn.cursor() cursor.execute("SELECT * FROM employees") for row in cursor.fetchall(): print(row) except cx_Oracle.DatabaseError as e: print(f"Database Error: {e}") finally: cursor.close() conn.close()
Best Practices for Connecting Python with Oracle
When integrating Python with Oracle, follow these best practices:
- Use Environment Variables – Store database credentials securely instead of hardcoding them in the script.
- Enable Connection Pooling – Reduces connection overhead for high-performance applications.
- Use Parameterized Queries – Prevents SQL injection and enhances security.
- Implement Logging – Maintain logs to track database interactions and errors.
- Close Connections Properly – Always close connections and cursors after execution to free up resources.
How Shiv Technolabs Can Help
If you’re looking to connect Oracle with Python for enterprise applications, Shiv Technolabs can assist you with expert Python development services. As a trusted Python development company UAE, we offer:
- Custom Python-Oracle Integration – Optimized solutions tailored to business needs.
- Secure Database Connectivity – Implementation of best security practices.
- Performance Optimization – Efficient connection pooling for large-scale applications.
- Expert Support – Dedicated Python developers to handle integration and maintenance.
For businesses looking to hire Python developers, our team ensures seamless Oracle database connectivity with secure and scalable solutions.
Conclusion
Integrating Python with Oracle Database opens doors to efficient data management, automation, and analytics. By leveraging cx_Oracle, developers can interact with Oracle databases effortlessly—whether for querying data, inserting records, or handling transactions.
Shiv Technolabs specializes in matchmaking app development and dating app development services, offering seamless database integration to ensure top-notch performance. Our expertise in connecting Oracle with Python enables businesses to leverage data-driven applications with efficiency and security.
For expert assistance in matchmaking Python with Oracle, Shiv Technolabs provides top-tier Python development services to help businesses achieve smooth integration and database management.
Looking to integrate Python with Oracle for your next project? Contact Shiv Technolabs today!
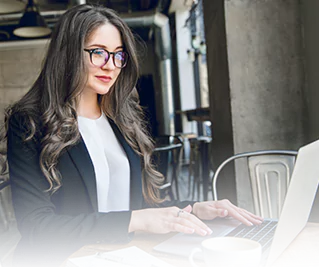