Table of Contents
JavaScript frameworks have become essential for building modern web applications. They provide a structured foundation, allowing developers to create scalable, efficient, and dynamic applications with less effort. In 2025, the JavaScript ecosystem continues to evolve, with frameworks driving innovations in both front-end and back-end development.
This blog covers the top 12 JavaScript frameworks that are dominating the development landscape in 2025. We’ll explore their key features, use cases, and code examples to help you decide which framework best fits your project needs.
What Is a JavaScript Framework?
A JavaScript framework is a pre-built collection of code that provides structure for developing web applications. Unlike libraries (which offer individual functions), frameworks define the overall architecture and dictate how your code should interact with the framework itself.
Frameworks provide:
- A defined structure for building applications.
- Pre-configured patterns for data binding, routing, state management, and rendering.
- Built-in tools to simplify complex development tasks like testing and code organization.
Frameworks are often divided into front-end development (handling user interfaces) and back-end development (handling server-side logic). Some frameworks, like Next.js and Nuxt.js, cover both front-end and back-end needs, making them full-stack solutions.
JavaScript Frameworks for Front-End
Front-end frameworks focus on building user interfaces and handling client-side interactions. They simplify the development of dynamic and responsive web pages by managing the DOM, state, and component rendering.
1. React.js – The Leading Front-End Framework
React.js, developed by Meta (Facebook), remains the most widely used JavaScript framework for building dynamic user interfaces. It introduced a component-based architecture and the concept of a virtual DOM to improve rendering efficiency.
Key Features:
- Component-based structure for reusable UI components.
- Virtual DOM for efficient updates.
- Strong ecosystem (Redux, React Router).
Use Case:
Ideal for single-page applications (SPAs) and real-time, data-driven user interfaces.
Example:
jsx
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return ( <div> <p>{count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }
2. Angular – Google’s Full-Fledged Framework
Angular, developed and maintained by Google, is a comprehensive framework using TypeScript. It follows an MVC (Model-View-Controller) architecture and includes powerful tools for dependency injection and state management.
Key Features:
- TypeScript-based framework.
- Built-in dependency injection.
- Strong CLI (Command Line Interface) for scaffolding and automation.
Use Case:
Suitable for large-scale enterprise applications with complex data flows and state management.
Example:
ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: `<h1>Hello {{ name }}!</h1>` }) export class AppComponent { name = 'Angular'; }
3. Vue.js – The Progressive Framework
Vue.js is known for its simplicity and flexibility. It combines the best features of React and Angular with a lightweight core and a straightforward templating system.
Key Features:
- Reactive data binding.
- Component-based architecture.
- Easy to integrate with existing projects.
Use Case:
Great for building SPAs and progressive web apps (PWAs).
Example:
vue
<template> <div> <p>{{ count }}</p> <button @click="count++">Increment</button> </div> </template> <script> export default { data() { return { count: 0 }; } }; </script>
4. Svelte – The Compiler-Based Game Changer
Svelte is a unique framework that shifts work from runtime to compile time. Instead of using a virtual DOM, Svelte compiles components into efficient JavaScript at build time.
Key Features:
- No virtual DOM – direct DOM manipulation.
- Small bundle size.
- Reactive state management without extra libraries.
Use Case:
Best for high-performance web applications where load speed and responsiveness are critical.
Example:
html
<script> let count = 0; </script> <button on:click={() => count++}> {count} </button>
Check this out: Popular React JS Frameworks
JavaScript Frameworks for Back-End
Back-end frameworks handle server-side logic, database management, and API creation. They provide the foundation for building scalable and secure server-side applications.
5. Express.js – The Lightweight Back-End Framework
Express.js is one of the most widely used JavaScript frameworks for building back-end applications with Node.js.
Key Features:
- Lightweight and fast.
- Middleware support for handling requests and responses.
- Strong routing capabilities.
Example:
js
const express = require('express'); const app = express(); app.get('/', (req, res) => { res.send('Hello from Express.js!'); }); app.listen(3000, () => { console.log('Server running on http://localhost:3000'); });
Must Read: Connect React to Express.js
6. Fastify – Fast and Low Overhead Back-End Framework
Fastify is a lightweight back-end framework focused on performance and low latency.
Key Features:
- High performance and low latency.
- Built-in schema-based validation.
Example:
js
CopyEdit
const fastify = require('fastify')(); fastify.get('/', async (request, reply) => { return { message: 'Hello from Fastify!' }; }); fastify.listen(3000);
7. NestJS – TypeScript-Based Back-End Framework
NestJS is a TypeScript-based framework for building scalable and maintainable server-side applications. Built on top of Node.js, it follows a modular architecture inspired by Angular and supports both RESTful APIs and GraphQL-based microservices.
Key Features:
- TypeScript support for static typing and better tooling.
- Built-in dependency injection.
- Modular architecture, similar to Angular.
- Powerful middleware support.
- Supports WebSockets and GraphQL out of the box.
Use Case:
Best for building enterprise-level APIs, microservices, and complex server-side applications requiring scalability and modularity.
Example:
ts
import { Controller, Get } from '@nestjs/common'; @Controller('hello') export class HelloController { @Get() getHello(): string { return 'Hello from NestJS!'; } }
Full-Stack JavaScript Frameworks
Full-stack frameworks handle both front-end and back-end development, providing a unified development environment.
8. Next.js – React-Based Full-Stack Framework
Next.js, developed by Vercel, extends React by adding server-side rendering (SSR), static site generation (SSG), and API routing. It allows developers to build highly performant applications that rank well on search engines.
Key Features:
- Hybrid rendering (supports both SSR and SSG).
- Built-in routing and API handling.
- Image optimization and automatic code splitting.
- Middleware support for handling requests and responses.
Use Case:
Best for SEO-optimized websites, content-heavy platforms, and dynamic web applications.
Example:
jsx
export default function Home() { return <h1>Welcome to Next.js!</h1>; }
9. Nuxt.js – Vue-Based Full-Stack Framework
Nuxt.js is the Vue.js equivalent of Next.js. It provides server-side rendering, static site generation, and client-side rendering options for Vue-based applications.
Key Features:
- Automatic routing based on file structure.
- Server-side rendering (SSR) and static site generation (SSG).
- Middleware and store integration.
- Built-in SEO optimization.
Use Case:
Best for building Vue-based SEO-friendly SPAs and static websites.
Example:
vue
<template> <div> <h1>{{ message }}</h1> </div> </template> <script> export default { data() { return { message: "Hello from Nuxt!" }; } }; </script>
10. Meteor.js – Full-Stack Real-Time Framework
Meteor.js is a full-stack JavaScript framework known for its real-time data handling capabilities. It allows developers to write both front-end and back-end code in the same environment.
Key Features:
- Real-time data synchronization.
- Built-in MongoDB support.
- Isomorphic code (same codebase for client and server).
- Large ecosystem of plugins and packages.
Use Case:
Best for building real-time chat apps, dashboards, and collaboration tools.
Example:
js
Meteor.methods({ 'tasks.insert'(text) { Tasks.insert({ text, createdAt: new Date() }); } });
11. Remix – Modern Full-Stack React Framework
Remix is a React-based framework focused on improving user experience through better data handling and rendering. It supports both client-side and server-side rendering.
Key Features:
- Built-in nested routing.
- Form handling and data submission.
- Enhanced SEO with server-side rendering.
- Supports data fetching at the component level.
Use Case:
Best for building data-driven applications and dynamic websites with complex routing needs.
Example:
jsx
import { useLoaderData } from "@remix-run/react"; export const loader = async () => { return { message: "Hello from Remix!" }; }; export default function Home() { const data = useLoaderData(); return <h1>{data.message}</h1>; }
12. Gatsby – Static Site Generator
Gatsby is a React-based static site generator that builds fast, SEO-friendly websites. It uses GraphQL for data fetching and generates static pages at build time.
Key Features:
- GraphQL-based data fetching.
- Pre-rendering for faster load times.
- Plugin support for extended functionality.
Use Case:
Best for blogs, portfolios, and documentation sites.
Example:
jsx
import React from "react"; export default function Home() { return <h1>Welcome to Gatsby!</h1>; }
Why JavaScript Frameworks Matter?
JavaScript remains the most popular programming language worldwide, according to the Stack Overflow Developer Survey. Frameworks streamline development by offering ready-to-use structures and patterns, which improves productivity and scalability.
# Key Benefits of Using JavaScript Frameworks:
- Faster Development: Pre-built components and templates speed up development.
- Scalability: Frameworks are designed to handle growing complexity and user demand.
- Performance: Many frameworks optimize rendering and data handling for faster load times.
- Cross-Platform: Some frameworks support web, mobile, and desktop development from a single codebase.
- Community Support: Popular frameworks have large communities and ecosystems, providing ready-made solutions for common challenges.
Why Choose Shiv Technolabs for JavaScript Development?
At Shiv Technolabs, we specialize in creating high-performance web applications using the latest JavaScript frameworks. Our expert developers have deep experience with front-end, back-end, and full-stack frameworks, ensuring that your project is built with the right tools for maximum scalability and performance.
Whether you need a dynamic user interface with React or Vue, a high-performance back-end with NestJS or Fastify, or a full-stack solution with Next.js or Nuxt.js — we’ve got you covered.
👉 Contact Shiv Technolabs today and let us transform your web development ideas into reality with cutting-edge JavaScript frameworks!
Which JavaScript Framework is Best for Your Business?
Choosing the right JavaScript framework depends on your business goals, project requirements, and technical needs. Here’s a quick guide to help you decide:
- Best for Fast Prototyping: Use Svelte or Vue.js for rapid development and a gentle learning curve.
- Best for Scalability: NestJS and Next.js are ideal for large-scale applications that require server-side rendering and modular architecture.
- Best for Real-Time Applications: Choose Meteor.js for real-time data synchronization and collaborative platforms.
- Best for SEO: Next.js, Nuxt.js, and Gatsby offer built-in SEO features and server-side rendering for better search engine rankings.
- Best for Performance: Fastify and Express.js are lightweight frameworks designed to handle high traffic with low latency.
- Best for Complex Front-End Applications: React and Angular are great for building complex, component-based UIs with state management.
Every framework has its strengths — understanding your project’s scale, complexity, and performance needs will help you select the most suitable option.
Conclusion
JavaScript frameworks continue to shape the future of web development in 2025. From front-end frameworks like React, Angular, and Vue to back-end solutions like Express and NestJS — the right framework can significantly improve your application’s performance, scalability, and user experience.
Understanding the strengths of each framework allows you to choose the best one for your project’s needs. Whether you’re building a small web app or a large-scale enterprise platform, JavaScript frameworks provide the flexibility and efficiency required to succeed in the competitive digital landscape.
Start building with the best JavaScript framework today and create the next big thing!
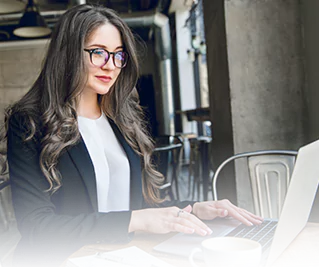