As digital transformation continues to reshape industries, cloud-based application development has become central to how businesses build, manage, and scale their digital services. Python has emerged as a popular choice for cloud-based applications due to its versatility, ease of use, and strong library support.
This guide will walk through the essential steps, strategies, and considerations for developing robust, cloud-based Python applications, covering everything from choosing the right platform to building scalable and secure solutions.
Introduction to Cloud-based Python Application Development
Cloud computing offers an infrastructure that lets developers run and manage applications without the need for in-house servers. This approach significantly reduces overhead, allowing developers to focus on innovation and agility. Python’s compatibility with cloud services and the flexibility it offers make it a preferred language for cloud-based applications. By using Python, developers can work with frameworks, libraries, and tools tailored to handle the unique challenges of the cloud environment, including data storage, security, and performance.
Key Components of Cloud-based Python Development
Building Python applications in the cloud requires a mix of knowledge in cloud infrastructure and Python-specific tools. Here are the primary components:
- Cloud Infrastructure: A strong foundation in cloud infrastructure (servers, databases, and networking) is essential. The main cloud providers—AWS, Google Cloud, and Azure—each offer unique services and architecture that impact the way Python applications are developed and run.
- Frameworks and Libraries: Python’s compatibility with a wide array of frameworks, such as Django and Flask, enables the creation of cloud-native applications. Additionally, libraries for handling database operations (like SQLAlchemy) and data processing (such as Pandas) are invaluable.
- Containers and Microservices: Containers like Docker and orchestration tools like Kubernetes are commonly used to deploy Python applications in a modular and scalable way. Microservices architecture, where applications are split into smaller, manageable services, allows for easier maintenance and faster scaling.
Choosing the Right Cloud Platform for Python Applications
Selecting the right cloud provider is a critical step. Each platform offers unique features, so it’s important to match the requirements of your Python application with the strengths of the cloud provider.
- Amazon Web Services (AWS): AWS is a leading choice for developers needing a range of services, from compute power to machine learning tools. With services like EC2, Lambda, and S3, AWS offers flexibility and scalability, especially for Python applications that demand high performance.
- Google Cloud Platform (GCP): Google Cloud provides strong data analysis tools and machine learning support, making it a good option for Python applications that work with large datasets or need real-time processing.
- Microsoft Azure: Known for its excellent integration with enterprise systems, Azure is ideal for businesses already using Microsoft products. Azure offers solid support for Python applications and has an extensive selection of services.
Building Scalable Python Applications in the Cloud
Scalability is crucial in cloud environments, where applications must handle varying loads and performance needs. Here are some essential practices for creating scalable cloud-based Python applications:
- Designing with Microservices: Adopting a microservices architecture allows applications to scale by dividing functionalities into smaller, independent services. Each service can scale independently, providing resilience and flexibility.
- Using Load Balancing: Implementing load balancers within the cloud environment distributes incoming traffic, maintaining performance levels. This is especially helpful when scaling up Python applications that may experience high traffic surges.
- Auto-scaling: Most cloud platforms offer auto-scaling services that add or remove resources based on demand. This feature is particularly beneficial for applications that experience variable traffic or seasonality, helping to manage costs and performance efficiently.
Serverless Computing with Python: An Overview
Serverless architecture is another way to manage and deploy cloud applications. In this model, the cloud provider automatically handles the infrastructure, letting developers focus on the application code.
- Benefits of Serverless Architecture: Serverless offers cost efficiency and agility, as there’s no need to maintain servers or scale manually. Services like AWS Lambda, Google Cloud Functions, and Azure Functions support Python, enabling developers to build functions that run in response to events.
- Setting Up Serverless Functions: Serverless functions are ideal for Python applications with modular tasks, such as API calls or event-driven actions. Functions can be designed to execute independently, allowing for rapid deployment and updating without affecting the larger application.
Data Management in Cloud-based Python Applications
Data storage and management are core aspects of cloud applications, as cloud platforms provide a range of data handling solutions tailored for different needs.
- Cloud Databases: Major cloud providers offer both SQL and NoSQL databases, such as AWS DynamoDB, Google Cloud Firestore, and Azure Cosmos DB. Python’s libraries like SQLAlchemy support easy interaction with these databases.
- Data Processing and Storage: Handling large datasets in Python applications often requires tools that can manage structured and unstructured data. Data lakes, like AWS Lake Formation or Google BigQuery, support large-scale data processing, ideal for applications with significant data needs.
- Security and Compliance: Ensuring data security is critical in cloud-based applications. Cloud providers offer encryption, role-based access, and data governance tools to protect sensitive data.
Setting Up Continuous Integration/Continuous Deployment (CI/CD)
Automation is key in cloud development, and CI/CD pipelines are essential for efficient, consistent deployment.
- Automated Testing and Deployment: CI/CD pipelines help maintain code quality by automating tests and deployments. Tools like GitHub Actions, Jenkins, and CircleCI support Python applications and enable a seamless deployment process.
- Code Quality and Version Control: Integrating code reviews and version control with tools like Git and GitLab ensures that teams can track changes and revert when necessary. CI/CD setups help deliver reliable applications, with the option to roll back changes if issues arise.
Enhancing Security for Cloud-based Python Applications
Security is a top priority in cloud environments, where applications face risks ranging from data breaches to DDoS attacks.
- Encryption and Data Privacy: Encrypting data both in transit and at rest is essential to prevent unauthorized access. Most cloud providers offer built-in encryption features to protect data integrity.
- Authentication and Authorization: Using secure identity management tools, such as AWS IAM or Azure Active Directory, helps regulate access to sensitive data and application functions, protecting against unauthorized access.
- Network Security: Setting up firewalls, VPNs, and network monitoring services on cloud platforms offers another layer of protection. Regular audits and vulnerability assessments further strengthen security.
Cost Management for Cloud-based Python Applications
Effective cloud cost management is essential for budget-friendly development.
- Understand Pricing Structures: Each cloud provider offers a different pricing model. Familiarity with compute time, data storage costs, and API call charges allows developers to estimate and control expenses effectively.
- Use Auto-scaling Features: Implementing auto-scaling not only supports performance but also helps control costs by adding resources only when needed. Setting thresholds for scaling prevents unnecessary charges.
- Monitor and Optimize Resources: Regularly reviewing cloud resources can help identify and eliminate underutilized services. Many providers offer budgeting tools and alerts to keep costs in check.
Leveraging AI and Machine Learning in Python Cloud Applications
Cloud platforms have transformed the ability to deploy AI and machine learning solutions at scale. Python’s ML libraries, combined with cloud services, make it possible to integrate intelligence into applications without extensive infrastructure.
- Popular Libraries: Libraries like TensorFlow, PyTorch, and scikit-learn offer frameworks for developing machine learning models. Many cloud providers have services that directly support these libraries, such as AWS SageMaker and Google AI Platform.
- Building and Deploying ML Models: Python applications can use ML models for a variety of tasks, such as data analysis, prediction, and pattern recognition. By deploying models in the cloud, developers can offer real-time insights and solutions.
- AI-Powered APIs: Many cloud providers offer APIs for language processing, computer vision, and predictive analytics. Integrating these APIs can accelerate development while reducing the need to build models from scratch.
# Future Trends in Cloud-based Python Development
The cloud continues to evolve, opening up new possibilities for Python developers:
- Edge Computing: Edge computing, which moves processing closer to data sources, is becoming increasingly popular for applications that require low-latency responses. Python’s lightweight nature makes it a good fit for edge devices.
- Quantum Computing: Some cloud providers are exploring quantum computing, a technology that promises breakthroughs in processing power. Python’s versatility means it could play a role in this emerging area as tools and libraries are developed.
- Hybrid and Multi-cloud Solutions: Many businesses are opting for hybrid solutions, combining public and private clouds to achieve flexibility. Python applications can easily integrate across multiple platforms, providing a cohesive experience.
Conclusion
Cloud-based Python application development provides a powerful toolkit for creating scalable, secure, and innovative applications. With the right cloud platform, architecture, and approach, developers can build solutions that meet the demands of modern users while keeping costs manageable. As cloud technology advances, Python’s role in cloud applications is likely to grow, making it an invaluable skill for developers.
In today’s fast-paced digital landscape, leveraging cloud-based Python applications can revolutionize business operations. If you’re seeking top-tier expertise in this field, Shiv Technolabs stands as a leading Python development company in UAE. Our team offers comprehensive Python development services in UAE, dedicated to building secure, scalable, and innovative cloud-based solutions tailored to your unique business needs. Partner with us to bring your vision to life and gain a competitive edge in the market.
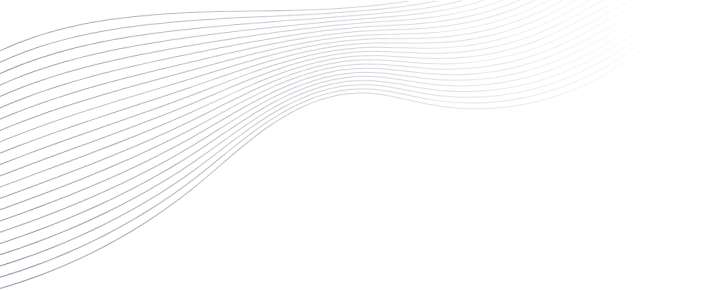
Revolutionize Your Digital Presence with Our Mobile & Web Development Service. Trusted Expertise, Innovation, and Success Guaranteed.