Adding a payment gateway to your Django e-commerce platform is a crucial step to allow customers to make secure transactions. This guide outlines the steps required to integrate a payment gateway into a Django project.
Step 1: Choose a Payment Gateway
Start by selecting a payment gateway that suits your business needs. Popular options include Stripe, PayPal, Razorpay, and Square. Evaluate them based on factors like pricing, support for currencies, geographic availability, and features.
Step 2: Install the Payment Gateway’s SDK or Library
Most payment gateways provide official Python SDKs or APIs to simplify integration. For example, if you are using Stripe, install its Python library:
pip install stripe
Similarly, check the official documentation of the chosen gateway for their specific installation steps.
Step 3: Configure Payment Gateway Credentials
After registering your e-commerce platform with the payment gateway, obtain the necessary credentials such as the API key, secret key, and client ID. Store these in your Django project’s settings file securely. Use Django’s os.environ for storing sensitive information.
Example for settings.py:
import os STRIPE_PUBLIC_KEY = os.getenv('STRIPE_PUBLIC_KEY') STRIPE_SECRET_KEY = os.getenv('STRIPE_SECRET_KEY')
Add these keys to your .env file:
STRIPE_PUBLIC_KEY=your_public_key STRIPE_SECRET_KEY=your_secret_key
Step 4: Create Payment Models
Define models to track payment-related data. This is essential for managing transaction records and verifying payment statuses.
Example models.py:
from django.db import models class Payment(models.Model): user = models.ForeignKey('auth.User', on_delete=models.CASCADE) amount = models.DecimalField(max_digits=10, decimal_places=2) transaction_id = models.CharField(max_length=100) payment_status = models.CharField(max_length=50, choices=[ ('Pending', 'Pending'), ('Completed', 'Completed'), ('Failed', 'Failed') ]) created_at = models.DateTimeField(auto_now_add=True) def __str__(self): return f'{self.user} - {self.amount} - {self.payment_status}'
Step 5: Set Up Payment Processing Logic
Write a view to handle the payment process. Use the payment gateway’s API to create a payment session or handle transactions.
Example for Stripe:
import stripe from django.conf import settings from django.http import JsonResponse stripe.api_key = settings.STRIPE_SECRET_KEY def create_checkout_session(request): try: session = stripe.checkout.Session.create( payment_method_types=['card'], line_items=[ { 'price_data': { 'currency': 'usd', 'product_data': { 'name': 'E-commerce Product', }, 'unit_amount': 5000, }, 'quantity': 1, }, ], mode='payment', success_url='https://yourdomain.com/success/', cancel_url='https://yourdomain.com/cancel/', ) return JsonResponse({'id': session.id}) except Exception as e: return JsonResponse({'error': str(e)})
Step 6: Set Up Webhooks for Payment Status Updates
Webhooks notify your platform about transaction updates like payment success or failure. Configure your Django application to handle webhook requests securely.
Example webhook view for Stripe:
from django.views.decorators.csrf import csrf_exempt from django.http import JsonResponse import stripe @csrf_exempt def stripe_webhook(request): payload = request.body sig_header = request.META['HTTP_STRIPE_SIGNATURE'] endpoint_secret = 'your_webhook_secret' try: event = stripe.Webhook.construct_event(payload, sig_header, endpoint_secret) except ValueError as e: return JsonResponse({'error': 'Invalid payload'}, status=400) except stripe.error.SignatureVerificationError as e: return JsonResponse({'error': 'Invalid signature'}, status=400) if event['type'] == 'checkout.session.completed': session = event['data']['object'] # Update your payment record or order status here return JsonResponse({'status': 'success'})
Step 7: Update Frontend for Payment Integration
Integrate the payment gateway’s checkout interface into your frontend. Many gateways offer hosted payment pages or client-side libraries to simplify this step. For Stripe, you can embed the checkout session ID into your JavaScript code:
const stripe = Stripe('your_public_key'); document.querySelector('#checkout-button').addEventListener('click', () => { fetch('/create-checkout-session/') .then(response => response.json()) .then(session => stripe.redirectToCheckout({ sessionId: session.id })) .catch(error => console.error('Error:', error)); });
Step 8: Test the Integration
Run tests in a sandbox or staging environment provided by the payment gateway. Simulate various scenarios like successful payments, declined cards, and network errors to confirm the integration handles all cases effectively.
Step 9: Enable Live Mode
Once testing is complete, switch the payment gateway to live mode. Update your API keys to the production credentials in your settings file.
Step 10: Monitor and Maintain
Continuously monitor payment transactions and maintain security updates for the payment gateway SDK or library. Regularly audit the payment process to address any potential vulnerabilities or issues.
Integrating a payment gateway in Django e-commerce platforms requires careful attention to security and functionality. Following these steps will help you set up a reliable and user-friendly payment system.
At Shiv Technolabs, we specialize in providing top-notch Python development services and tailored solutions for businesses of all sizes. Whether you’re looking to integrate payment gateways, build custom e-commerce platforms, or enhance your existing applications, our expert team is here to help. When you hire Django developers from Shiv Technolabs, you gain access to skilled professionals dedicated to delivering robust, secure, and scalable applications. We also offer Python development services in Australia, ensuring high-quality solutions tailored to your business needs. Let us turn your vision into reality with cutting-edge Django solutions designed to drive your business forward. Reach out today to get started!
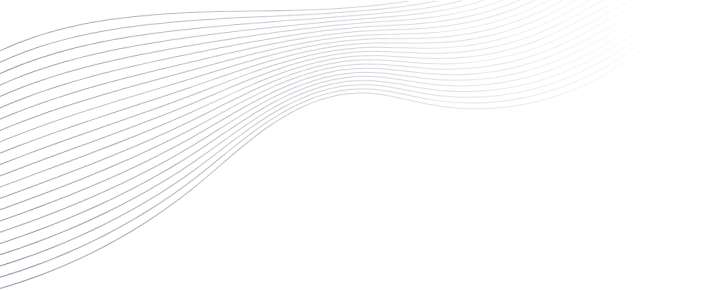
Revolutionize Your Digital Presence with Our Mobile & Web Development Service. Trusted Expertise, Innovation, and Success Guaranteed.