Table of Contents
Building a secure NodeJS REST API framework is crucial for modern web applications. In this guide, we’ll cover how to create API in Node JS Express, a popular framework for building RESTful services. Express provides a minimalistic approach to API development, making it a favorite among developers for its simplicity and flexibility.
To start, we’ll set up a basic NodeJS REST API framework with essential security measures. We’ll use Express to handle routing and middleware, ensuring that our API is robust and efficient. Proper Node JS REST API documentation is also essential for maintaining and scaling your application. We’ll include steps for documenting your API endpoints to make it easier for other developers to understand and contribute to your project.
Also Read:- NodeJS Development: A Complete Guide
For those seeking expert assistance, a Node.js development company can provide specialized services to enhance your API’s performance and security. By following this guide, you’ll learn how to quickly and securely set up a NodeJS REST API, making use of Express for rapid development and efficient handling of HTTP requests.
Node.js REST API Setup in 5 Minutes
Building a secure Node.js REST API quickly is crucial in today’s fast-paced development environment. This guide will walk you through the steps to set up a secure Node.js REST API in just five minutes, covering essential security measures to protect your application from common vulnerabilities.
Step 1: Initial Setup
First, ensure you have Node.js and npm installed on your machine. You can verify the installation by running:
node -v npm -v
Next, create a new directory for your project and navigate into it:
mkdir secure-node-api cd secure-node-api
Initialize a new Node.js project with:
npm init -y
This will create a ‘package.json‘ file with default settings.
Step 2: Install Essential Packages
Install the necessary packages for your REST API, including Express for handling HTTP requests, and additional security-related packages:
npm install express helmet cors dotenv
- Express: A minimal and flexible Node.js web application framework.
- Helmet: Helps secure your Express apps by setting various HTTP headers.
- CORS: Middleware to enable Cross-Origin Resource Sharing.
- Dotenv: Loads environment variables from a ‘.env’ file into ‘process.env’.
Step 3: Basic Server Setup
Create a file named ‘server.js‘ and add the following code to set up a basic Express server:
const express = require('express'); const helmet = require('helmet'); const cors = require('cors'); require('dotenv').config(); const app = express(); const port = process.env.PORT || 3000; // Middleware app.use(helmet()); // Adds security headers app.use(cors()); // Enables CORS app.use(express.json()); // Parses JSON bodies // Sample route app.get('/', (req, res) => { res.send('Welcome to the secure Node.js REST API!'); }); app.listen(port, () => { console.log(`Server running on port ${port}`); });
Step 4: Secure Environment Variables
Create a ‘.env‘ file in the root directory to store sensitive information like your API keys and database credentials:
PORT=3000
Using environment variables helps keep sensitive data out of your source code.
Step 5: Implementing Basic Authentication
For basic authentication, we will use JWT (JSON Web Tokens). Install the necessary package:
npm install jsonwebtoken bcryptjs
- jsonwebtoken: Used to sign and verify tokens.
- bcryptjs: A library to hash passwords.
Step 6: User Authentication
Create a ‘user.js‘ file to handle user registration and login:
const express = require('express'); const jwt = require('jsonwebtoken'); const bcrypt = require('bcryptjs'); const router = express.Router(); const users = []; // In-memory user storage, replace with database in production // Register route router.post('/register', async (req, res) => { const { username, password } = req.body; // Hash the password const hashedPassword = await bcrypt.hash(password, 10); // Store user users.push({ username, password: hashedPassword }); res.status(201).send('User registered'); }); // Login route router.post('/login', async (req, res) => { const { username, password } = req.body; // Find user const user = users.find(u => u.username === username); if (!user) { return res.status(400).send('Invalid credentials'); } // Check password const isPasswordValid = await bcrypt.compare(password, user.password); if (!isPasswordValid) { return res.status(400).send('Invalid credentials'); } // Generate token const token = jwt.sign({ username: user.username }, process.env.JWT_SECRET, { expiresIn: '1h' }); res.send({ token }); }); module.exports = router;
Step 7: Protecting Routes
Protect routes by verifying the JWT token. Create a middleware for token verification:
const jwt = require('jsonwebtoken'); function authenticateToken(req, res, next) { const authHeader = req.headers['authorization']; const token = authHeader && authHeader.split(' ')[1]; if (!token) { return res.sendStatus(401); } jwt.verify(token, process.env.JWT_SECRET, (err, user) => { if (err) { return res.sendStatus(403); } req.user = user; next(); }); } module.exports = authenticateToken;
In ‘server.js‘, use this middleware to protect routes:
const express = require('express'); const helmet = require('helmet'); const cors = require('cors'); const dotenv = require('dotenv'); const userRoutes = require('./user'); const authenticateToken = require('./middleware/authenticateToken'); dotenv.config(); const app = express(); const port = process.env.PORT || 3000; // Middleware app.use(helmet()); app.use(cors()); app.use(express.json()); // Public routes app.use('/api/user', userRoutes); // Protected route app.get('/api/protected', authenticateToken, (req, res) => { res.send('This is a protected route'); }); app.listen(port, () => { console.log(`Server running on port ${port}`); });
Your Turn
In conclusion, setting up a secure NodeJS REST API framework is essential for developing reliable and scalable web applications. By following the steps outlined in this guide, you now have a foundational understanding of how to create API in Node JS Express, implement basic security measures, and maintain comprehensive Node JS REST API documentation. This ensures your API is well-structured, secure, and easy to understand for both current and future developers.
For those looking to take their API development to the next level, seeking professional assistance can be a game-changer. Shiv Technolabs offers top-notch Node.js development services Australia, specializing in creating secure, efficient, and scalable APIs. Our team of experts is dedicated to helping you build robust applications that meet your business needs. Partner with Shiv Technolabs to ensure your Node.js projects are handled with the utmost expertise and care.
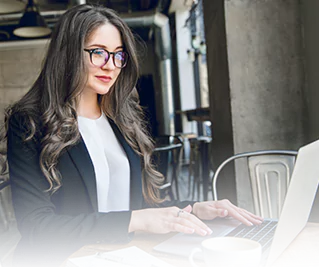