Table of Contents
APIs, or Application Programming Interfaces, are vital in modern web development, allowing different software systems to communicate and share data efficiently. In Laravel, APIs can be created effortlessly, leveraging the framework’s rich features to build efficient and scalable solutions. Whether it’s a RESTful API or a GraphQL API, Laravel provides the necessary tools to integrate and secure these interfaces effectively. This article will discuss the types of APIs in Laravel and the importance of security in API integrations before detailing the steps to implement secure API integrations in Laravel.
APIs have become a cornerstone of web development, with over 90% of developers reporting the use of APIs in their applications according to a recent survey by Postman. The same survey highlighted that 55% of APIs are developed with a focus on security, demonstrating the increasing importance of secure API practices. For companies offering Laravel website development services in Australia, ensuring API security is crucial to protect sensitive data and maintain client trust. By using Laravel’s built-in tools and following best practices, developers can build robust and secure APIs that meet the demands of modern web applications.
Laravel’s popularity continues to rise, with over 700,000 websites worldwide utilizing the framework, as reported by BuiltWith. In Australia, backend development companies are witnessing growing demand due to the framework’s flexibility and comprehensive feature set. For dedicated Laravel developers, the ability to build secure APIs is a significant advantage, as secure integrations are essential for e-commerce platforms, financial services, and other industries handling sensitive information. Effective API security not only protects data but also enhances the overall performance and reliability of web applications, making Laravel a preferred choice for businesses and developers alike.
What are APIs and How Do They Work in Laravel?
APIs enable different software applications to interact using defined protocols and tools. In Laravel, APIs function by handling HTTP requests and returning responses, facilitating operations like data retrieval, updating, and deletion. Laravel’s expressive syntax and comprehensive tools make it ideal for creating robust APIs. With features like Eloquent ORM, middleware, and built-in support for API routes, Laravel simplifies the process of building and managing APIs.
Types of APIs in Laravel (RESTful, GraphQL, etc.)
Laravel supports various types of APIs, each with specific use cases and benefits. The most common types include:
- RESTful APIs: These APIs follow the principles of Representational State Transfer (REST), using HTTP methods such as GET, POST, PUT, and DELETE to perform CRUD operations. RESTful APIs are stateless and use standard HTTP status codes and conventions, making them easy to use and integrate.
- GraphQL APIs: GraphQL, developed by Facebook, allows clients to request exactly the data they need, reducing over-fetching and under-fetching of data. Laravel provides support for GraphQL through packages like Lighthouse, enabling developers to build flexible and efficient APIs.
Each type of API has its advantages, and the choice depends on the specific requirements of the application and the preferences of the development team.
Also read : Laravel Web Development Benefits for US Businesses
Importance of Security in API Integrations
Security is critical in API integrations, as APIs often handle sensitive data and perform essential operations. Without proper security measures, APIs can become vulnerable to attacks such as SQL injection, cross-site scripting (XSS), and man-in-the-middle attacks. Ensuring that APIs are secure protects both the data and the integrity of the application, preventing unauthorized access and data breaches. For businesses offering Laravel website development services in Australia, maintaining high security standards in API development is essential to build trust and comply with data protection regulations.
Steps to Implement Secure API Integrations in Laravel
1) Use Environment Variables
Storing sensitive information such as API keys and secrets in environment variables is a fundamental security practice in Laravel. By placing these variables in the .env file, you protect them from exposure in the codebase or version control systems.
- Storing API Keys: Store API keys, database credentials, and other sensitive information in the .env file. This practice prevents them from being hardcoded in your application’s source code.
- Accessing Environment Variables: Use the env() function to retrieve these values within your application. For example, env(‘API_KEY’) allows you to access the API key stored in the environment variable
2) Secure API Requests
Ensuring that all API requests are secure is crucial for protecting data in transit. This involves using HTTPS and validating SSL certificates.
- Enforcing HTTPS: Always use HTTPS for API requests to encrypt data between the client and the server. This measure prevents data from being intercepted and read by unauthorized parties.
- Validating SSL Certificates: Ensure that SSL certificates are valid and correctly configured. This adds an extra layer of security, verifying the identity of the server and encrypting the data.
3) Implement Authentication
Authentication verifies the identity of users or systems interacting with the API. Laravel offers various methods for implementing authentication.
- OAuth Implementation: OAuth is a widely used protocol for token-based authentication, allowing users to grant third-party access to their resources without sharing credentials. Laravel supports OAuth through the Laravel Passport package.
- Using JWT Tokens: JSON Web Tokens (JWT) are another method for token-based authentication. Laravel provides JWT support through packages like jwt-auth.
- Securing API Tokens: Store API tokens securely and avoid exposing them in URLs or logs. Use headers for token transmission.
4) Rate Limiting
Rate limiting controls the number of requests a client can make to the API within a specific timeframe, preventing abuse and ensuring fair usage.
- ThrottleRequests Middleware: Laravel’s ThrottleRequests middleware allows you to set rate limits for your API endpoints. Configure the middleware to define the maximum number of requests and the timeframe.
5) Input Validation and Sanitization
Validating and sanitizing inputs prevents malicious data from compromising your application. Laravel offers robust validation mechanisms to ensure data integrity.
- Preventing SQL Injection: Use Laravel’s Eloquent ORM or Query Builder to interact with the database, which automatically escapes inputs to prevent SQL injection attacks..
- Ensuring Data Integrity: Validate inputs using Laravel’s built-in validation rules. Sanitize data to remove harmful content before processing.
6) Error Handling
Proper error handling ensures that sensitive information is not exposed in error messages and logs.
- Using Try-Catch Blocks: Wrap code that may throw exceptions in try-catch blocks to handle errors gracefully. Return appropriate HTTP status codes and messages to the client..
- Logging Errors Safely: Use Laravel’s logging system to log errors without exposing sensitive information. Configure logging channels and levels in the logging.php configuration file.
7) Data Encryption
Encrypting sensitive data before storing or transmitting it protects against unauthorized access.
- Encrypting Sensitive Data: Use Laravel’s built-in encryption functions to encrypt data. For example, Crypt::encrypt($data) and Crypt::decrypt($encryptedData) can be used to encrypt and decrypt data.
- Laravel’s Built-In Encryption Functions: Leverage Laravel’s encryption capabilities to secure data at rest and in transit.’
8) Use Laravel HTTP Client
Laravel’s HTTP client provides a simple and secure way to make API requests, with built-in support for common security features.
- Benefits of Laravel HTTP Client: The HTTP client, introduced in Laravel 7, offers a fluent interface for making HTTP requests, handling responses, and managing errors.
- Secure API Requests: Use the HTTP client to send secure API requests, automatically handling SSL verification and other security aspects.
9) Set Up CORS Policies
Cross-Origin Resource Sharing (CORS) policies control which domains can access your API, enhancing security by restricting access.
- Controlling Access with CORS: Define CORS policies to specify allowed origins, methods, and headers. This prevents unauthorized domains from accessing your API.
- Implementing Cors Middleware: Use Laravel’s Cors middleware to enforce CORS policies. Configure the middleware in the cors.php configuration file.
10) Regular Security Audits
Conducting regular security audits helps identify and address vulnerabilities in your API.
- Conducting Security Audits: Perform periodic security audits to assess the security of your API and identify potential weaknesses.
- Keeping Dependencies Updated: Regularly update Laravel and its dependencies to have the latest security patches and features.
11) Limit Data Exposure
Minimizing data exposure in API responses reduces the risk of sensitive information being leaked.
- Shaping Data with Resource Classes: Use Laravel’s resource classes to control the data structure in API responses, exposing only the necessary information.
- Minimizing Data in Responses: Avoid including unnecessary data in API responses. Use techniques like sparse fieldsets to limit the data returned to the client.
12) API Rate Limiting and Throttling
Protecting your API from abuse involves implementing rate limiting and throttling to control the flow of requests.
- Implementing Rate Limits: Set rate limits for different API endpoints based on usage patterns and requirements. Use Laravel’s rate limiting features to enforce these limits.
- Protecting Your API: Throttling helps protect your API from being overwhelmed by too many requests, ensuring reliable performance.
13) Versioning the API
API versioning allows you to manage changes and deprecations without disrupting existing clients.
- Managing API Changes: Implement versioning to introduce changes and new features without breaking existing clients. Use URI versioning (e.g., /api/v1/resource) or header versioning.’
- Implementing API Versioning: Plan and communicate versioning strategies to your clients, ensuring a smooth transition between API versions.
14) Logging and Monitoring
Logging and monitoring API activity helps track usage, identify issues, and ensure optimal performance.
- Monitoring API Usage: Use logging tools to monitor API requests, responses, and errors. Analyze logs to identify patterns and potential issues.
- Using Laravel Telescope: Laravel Telescope provides real-time insights into your application, including API requests, database queries, and exceptions. Use Telescope to monitor and debug your API.
15) CSRF Protection
Cross-Site Request Forgery (CSRF) protection prevents unauthorized actions being performed on behalf of authenticated users.
- Enabling CSRF Protection: Use Laravel’s built-in CSRF protection for forms and state-changing endpoints. CSRF tokens ensure that requests are legitimate.
- Protecting Forms and Endpoints: Automatically include CSRF tokens in forms and verify them in the backend. Use the VerifyCsrfToken middleware to enforce CSRF protection.
Conclusion
Implementing secure API integrations in Laravel involves a series of best practices and techniques to protect your application and data. From using environment variables to encrypting data and setting up CORS policies, each step enhances the security of your API. By following these guidelines, you can build secure and reliable APIs with Laravel, protecting both your application and its users.
For businesses in Australia looking for expert Laravel website development services, Shiv Technolabs is a leading Laravel web development company. Our dedicated Laravel developers specialize in Laravel API development, offering complete solutions to build secure and scalable APIs. Whether you need to build an API with Laravel or enhance your existing Laravel development projects, Shiv Technolabs has the expertise to deliver outstanding results. Partner with us to make the most of Laravel and ensure the success of your web development initiatives.
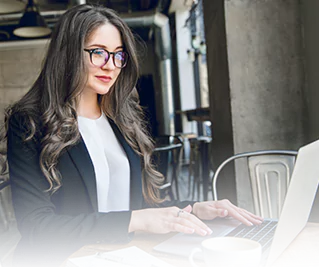