Laravel aims to make the development process more pleasant for developers by providing elegant syntax and reducing repetitive tasks. It offers a rich ecosystem of tools and libraries that cater to various aspects of web application development, from routing and templating to authentication and testing.
“As of 2024, Laravel powers approximately 1,723,068 websites globally. This includes both live and historical sites, demonstrating its widespread adoption and versatility in various industries.”
How Does Laravel Work?
Laravel operates on a foundation of simplicity and elegance. Here’s a high-level overview of how it works:
- Routing: Laravel’s routing system allows developers to define URL patterns and map them to specific controller actions. This makes it easy to handle incoming requests and direct them to the appropriate logic.
- Controllers and Middleware: Controllers handle the logic for specific routes. Middleware can be used to filter HTTP requests and perform tasks such as authentication and input validation.
- Views and Blade Templating: Laravel uses the Blade templating engine to create dynamic views. Blade allows developers to write concise, readable templates and includes features like template inheritance and data binding.
- Models and Eloquent ORM: The Eloquent ORM (Object-Relational Mapping) provides a simple, fluent interface for interacting with the database. Models represent database tables and allow developers to perform CRUD (Create, Read, Update, Delete) operations easily.
- Database Migration and Seeding: Laravel’s migration system helps manage database schema changes, while seeding allows developers to populate the database with initial data.
- Authentication and Authorization: Laravel includes built-in tools for user authentication and authorization, making it straightforward to implement secure access control.
- Artisan Console: Artisan is Laravel’s command-line interface, offering numerous commands to streamline common development tasks, such as database migrations, testing, and generating boilerplate code.
- Packages and Libraries: Laravel has a vibrant ecosystem of packages and libraries that extend its functionality. These packages can be easily integrated into projects to add features like payment processing, social media integration, and more.
Also Read:- Steps to Implement Secure API Integrations in Laravel
Laravel Web Application Development Process
The process of developing a web application using Laravel is structured and efficient, enabling Laravel developers to create robust, scalable, and feature-rich applications. Here, we will outline the detailed steps involved in the Laravel web application development process.
# Requirement Analysis
Before diving into development, it’s crucial to thoroughly understand the client’s needs and project requirements. This phase involves:
- Gathering Requirements: Detailed discussions with stakeholders to understand the application’s purpose, target audience, and key features.
- Defining Objectives: Setting clear, measurable goals for what the application should achieve.
- Scope and Constraints: Identifying any limitations or specific constraints related to technology, budget, or timeline.
# Planning and Design
With a clear understanding of the requirements, the next step is to plan and design the application. This phase includes:
- Architecture Planning: Designing the application’s architecture to ensure a solid foundation. This includes deciding on the MVC (Model-View-Controller) structure.
- Wireframes and Prototypes: Creating wireframes to visualize the application’s layout and user interface. Prototyping tools can help in building interactive prototypes for better understanding.
- Database Design: Defining the database schema, including tables, relationships, and data flow.
# Environment Setup
Setting up the development environment is critical for smooth development. This involves:
- Installing Laravel: Using Composer to install Laravel and its dependencies.
- Configuring Development Environment: Setting up environment variables and configuration files to match the development setup.
- Version Control: Initializing a version control system (e.g., Git) to manage code changes and collaboration.
# Database Management
Laravel provides powerful tools for database management, including:
- Migrations: Creating migration files to manage database schema changes. Migrations allow developers to version control the database schema and apply changes incrementally.
- Seeding: Using seeders to populate the database with initial data for testing and development purposes.
- Eloquent ORM: Leveraging Eloquent ORM for database interactions, enabling developers to work with database records using an object-oriented syntax.
# Feature Implementation
The core development phase involves implementing the application’s features, which includes:
- Routing: Defining routes to handle incoming requests and map them to appropriate controller actions.
- Controllers and Middleware: Writing controller methods to handle business logic and using middleware for tasks such as authentication and request filtering.
- Views and Blade Templating: Creating dynamic views using Blade templating engine, allowing for clean and reusable templates.
- Models and Eloquent ORM: Defining models to interact with the database and perform CRUD operations.
# Authentication and Authorization
Laravel provides built-in tools for authentication and authorization, making it easy to implement secure access control:
- User Authentication: Setting up user registration, login, and password reset functionalities using Laravel’s authentication scaffolding.
- Authorization: Defining roles and permissions to control access to various parts of the application.
# Testing
Testing is an integral part of the development process to ensure the application functions as expected:
- Unit Testing: Writing unit tests to test individual components and ensure they work correctly in isolation.
- Feature Testing: Testing the application’s features end-to-end to validate that they function as expected.
- Browser Testing: Using tools like Laravel Dusk to perform browser automation and test user interactions.
# Deployment
Once the application is developed and tested, it’s time to deploy it to a production environment:
- Server Configuration: Setting up the server, including web server (e.g., Apache, Nginx) and PHP configurations.
- Deployment Tools: Using deployment tools like Laravel Forge or Envoyer to automate the deployment process.
- Database Migration: Running database migrations on the production server to ensure the database schema is up-to-date.
# Maintenance and Support
After deployment, the application requires ongoing maintenance and support to ensure it continues to run smoothly:
- Bug Fixes: Monitoring for bugs and issues reported by users and addressing them promptly.
- Performance Optimization: Continuously optimizing the application’s performance to handle increasing traffic and data.
- Feature Enhancements: Adding new features and improvements based on user feedback and changing requirements.
- Security Updates: Applying security patches and updates to keep the application secure.
Also Read:- Introducing the Laravel Forge CLI: Streamlining Your Deployment Process
Why Choose Laravel for Web App Development?
There are several compelling reasons to choose Laravel for your web application development needs:
- Ease of Use: Laravel’s intuitive syntax and comprehensive documentation make it accessible to both beginners and experienced developers. Its straightforward learning curve allows developers to start building applications quickly.
- Rich Ecosystem: Laravel’s extensive ecosystem includes packages and tools for almost every aspect of web development. From authentication and API handling to task scheduling and caching, Laravel has you covered.
- Security: Laravel takes security seriously and includes features like CSRF (Cross-Site Request Forgery) protection, encryption, and secure password hashing out of the box. This helps safeguard your application from common security vulnerabilities.
- Scalability: Laravel’s modular architecture allows developers to build scalable applications. Whether you’re developing a small blog or a large enterprise system, Laravel can handle the load.
- Community and Support: Laravel boasts a large and active community of developers. This means you can find a wealth of resources, tutorials, and forums to help you troubleshoot issues and stay up to date with best practices.
- Testing: Laravel includes built-in support for testing with PHPUnit. This makes it easy to write and run tests, ensuring the reliability and stability of your application.
Use of Laravel Development Process for Web Solutions
The Laravel development process involves several key steps to ensure efficient and effective web solutions. Here’s a typical workflow:
- Requirement Analysis: Understanding the client’s needs and project requirements is the first step. This involves gathering information about the desired features, functionality, and user experience.
- Planning and Design: Once the requirements are clear, the next step is to plan the architecture and design the application. This includes creating wireframes, defining the database schema, and outlining the application’s structure.
- Development: The actual development process begins with setting up the Laravel environment and configuring the necessary dependencies. Developers then start building the application’s features, following best practices and coding standards.
- Database Management: Laravel’s migration system is used to manage database schema changes. Migrations allow developers to version control the database schema and make modifications without affecting existing data.
- Feature Implementation: Developers implement the core features of the application, such as user authentication, data processing, and user interfaces. This involves writing controllers, models, and views, as well as integrating third-party packages as needed.
- Testing: Testing is an integral part of the development process. Laravel’s testing framework allows developers to write unit tests, feature tests, and browser tests to ensure the application’s functionality and reliability.
- Deployment: Once the application is thoroughly tested and reviewed, it is deployed to a production environment. This involves configuring the server, setting up databases, and ensuring the application runs smoothly in a live setting.
- Maintenance and Support: After deployment, the application requires ongoing maintenance and support. This includes monitoring for bugs, applying security updates, and adding new features as needed.
Also Read:- 5 Programming Languages For Creating eCommerce Websites
Advantages of Laravel Development for Your Business
Choosing Laravel for web application development offers numerous benefits for businesses:
- Cost-Effective: Laravel’s open-source nature and extensive library of pre-built components reduce development time and costs. Businesses can achieve their goals without breaking the bank.
- Customization: Laravel allows for highly customizable solutions. Businesses can tailor the application to meet their specific needs and requirements, ensuring a unique and tailored experience for users.
- Quick Development: With its ready-to-use tools and streamlined development process, Laravel enables faster time-to-market. This agility is crucial in today’s competitive business landscape.
- Performance: Laravel is optimized for performance, ensuring that applications run efficiently and handle high traffic loads. This is essential for providing a seamless user experience.
- Integration Capabilities: Laravel can integrate with various third-party services and APIs, allowing businesses to extend the functionality of their applications easily. Whether it’s payment gateways, social media, or email services, Laravel can handle it.
- Scalability: As your business grows, your application can scale with it. Laravel’s modular architecture and support for caching, queueing, and database optimization ensure that your application can handle increased demand.
- Security: Security is a top priority in web development, and Laravel provides robust security features to protect sensitive data and prevent unauthorized access.
- Community Support: The Laravel community is a valuable resource for businesses. Whether you need help troubleshooting an issue or want to stay updated with the latest trends, the community is always there to support you.
Frequently Asked Question (FAQs)
1) What is Laravel, and why is it popular?
Laravel is a PHP framework designed for web application development. It is popular because of its elegant syntax, comprehensive documentation, and extensive ecosystem of tools and packages that simplify the development process.
2) Can Laravel be used for ERP web application development?
Yes, Laravel can develop ERP web applications. Its scalability, customization options, and robust security features make it an excellent choice for building complex, enterprise-level applications.
3) What are custom Laravel web application development services?
Custom Laravel web application development services involve creating tailored solutions to meet specific business needs. This includes developing unique features, integrating third-party services, and ensuring the application aligns with the business’s goals.
4) What tools are available for Laravel web application development?
Laravel offers a range of tools, including Artisan CLI for command-line tasks, Eloquent ORM for database management, Blade for templating, and various packages for authentication, caching, and more.
5) How do I choose a Laravel web application development company?
When choosing a Laravel web application development company, consider their experience, portfolio, client reviews, and expertise in Laravel. It’s essential to select a company that understands your business needs and can deliver a high-quality solution.
6) Is Laravel suitable for small businesses?
Yes, Laravel is suitable for small businesses. Its cost-effectiveness, ease of use, and scalability make it an ideal choice for businesses of all sizes.
7) What are the benefits of using Laravel for web app development?
Benefits of using Laravel include faster development, robust security features, scalability, customization options, and strong community support. It allows businesses to create high-performing, secure, and feature-rich applications.
The Laravel web application development process is comprehensive and well-structured, ensuring that applications are built efficiently and effectively. By following these steps, developers can create robust, scalable, and feature-rich applications that meet the needs of businesses and users alike.
Working with a skilled Laravel web application development company can further enhance the development process, providing expertise and resources to develop ERP web applications and other custom solutions. With the right approach and tools, Laravel can deliver powerful web applications that drive business success.
For businesses in Singapore looking to leverage Laravel’s capabilities, there are specialized Laravel development services available. These services can help tailor web applications to meet specific business needs, ensuring that you get the most out of your investment in Laravel web application development tools. Whether you need a simple website or a complex ERP system, custom Laravel web application development services can provide the solutions you need to stay competitive in the digital landscape.
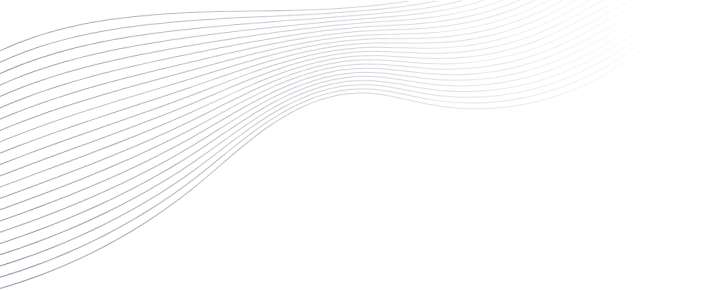
Revolutionize Your Digital Presence with Our Mobile & Web Development Service. Trusted Expertise, Innovation, and Success Guaranteed.