Table of Contents
Released on February 24, 2025, Laravel 12 marks a significant step forward for the popular PHP framework. This latest version focuses on enhancing security, performance, and the developer experience, all while maintaining minimal breaking changes.
Laravel 12 introduces a host of new features and improvements – from streamlined front-end scaffolding and robust API development enhancements to powerful debugging tools and performance optimizations.
In this in-depth overview, we’ll explore the key features and updates in Laravel 12 that every developer and business should know about.
New Starter Kits for React, Vue & Livewire
One of the major highlights of Laravel 12 is the introduction of new starter kits for React, Vue, and Livewire. These kits offer pre-configured front-end scaffolding and authentication, making it easier to set up modern applications.
The React and Vue starter kits come with Inertia 2, TypeScript support, and Tailwind CSS pre-configured. For Livewire, the new starter kit includes FluxUI, a Tailwind-based component library, and Laravel Volt for a seamless full-stack experience.
WorkOS AuthKit is also integrated into the starter kits, providing enterprise-grade authentication features such as social logins (Google, Facebook, etc.), passwordless authentication with passkeys, and single sign-on (SSO) support.
With the release of these new kits, Laravel Breeze and Jetstream are being deprecated. The new kits simplify the setup process and provide a modern development environment out of the box.
Enhanced Application Structure and Organization
Laravel 12 introduces a more refined and intuitive application structure to improve project organization.
The directory layout and configuration files have been streamlined, making it easier for developers to navigate large codebases. Configuration files are now more centralized, reducing clutter and improving consistency in how files and settings are organized.
This improved structure helps developers onboard faster and maintain codebases more effectively. As projects grow in complexity, this cleaner structure ensures that the code remains manageable and scalable.
Authentication and Security Enhancements
Security has always been a key focus for Laravel, and version 12 strengthens it further with improvements to authentication and session handling.
The new secureValidate() method simplifies the process of enforcing stronger password rules. Instead of manually defining complex validation rules, developers can use the strong keyword to enforce a higher password complexity.
Before (Laravel 11):
php
$request->validate([ 'password' => 'required|min:8', ]);
After (Laravel 12):
php
$request->secureValidate([ 'password' => ['required', 'min:8', 'strong'], ]);
This method ensures that passwords meet modern security standards, reducing the risk of weak password vulnerabilities.
Laravel 12 also improves session handling and OAuth support, making it easier to manage user authentication across multiple platforms. API authentication with Laravel Sanctum and Passport is more straightforward, ensuring secure handling of tokens and user sessions.
Advanced Query Builder and Eloquent Improvements
The query builder and Eloquent ORM receive significant upgrades in Laravel 12, making database interactions more powerful and efficient.
# Simpler Nested Where Clauses
The new nestedWhere() method simplifies complex queries that involve mixed AND/OR logic.
Before (Laravel 11):
php
$users = DB::table('users') ->where('status', 'active') ->where(function($query) { $query->where('age', '>', 25) ->orWhere('city', 'New York'); }) ->get();
After (Laravel 12):
php
$users = DB::table('users') ->where('status', 'active') ->nestedWhere('age', '>', 25, 'or', 'city', 'New York') ->get();
The new method makes the code cleaner and more readable while reducing the need for closures.
# Conditional Eager Loading with withFiltered
The withFiltered() method allows developers to load related models with specific conditions directly, simplifying relationship constraints.
Before (Laravel 11):
php
$users = User::with(['posts' => function($query) { $query->where('status', 'published'); }])->get();
After (Laravel 12):
php
$users = User::withFiltered('posts', ['status' => 'published'])->get(); These improvements reduce boilerplate code and make queries cleaner and more readable.
Improved API Development (GraphQL & Versioning)
Laravel 12 introduces native support for GraphQL and improves API versioning to simplify modern API development.
# GraphQL Support
With GraphQL support built into the framework, developers can now define GraphQL schemas and resolvers directly within Laravel. This allows clients to request only the data they need, reducing over-fetching and under-fetching issues common with REST APIs.
# Cleaner API Versioning
API versioning has also been streamlined with the new Route::apiVersion() method.
Before (Laravel 11):
php
Route::get('/api/v1/users', [UserController::class, 'index']); Route::get('/api/v2/users', [UserController::class, 'indexV2']);
After (Laravel 12):
php
Route::apiVersion(1)->group(function () { Route::get('users', [UserController::class, 'index']); });
This keeps route files well-organized and makes it easier to manage multiple API versions as the application evolves.
Performance and Scalability Improvements
Laravel 12 includes several under-the-hood improvements to boost performance and scalability.
# Asynchronous Caching
The framework now supports asynchronous cache operations, reducing response times by handling caching in the background.
# Improved Job Queue Handling
Laravel 12 improves the queue system with dynamic prioritization.
Before (Laravel 11):
php
dispatch(new ProcessOrder($order))->onQueue('high');
After (Laravel 12):
php
dispatch(new ProcessOrder($order))->prioritize('high');
These improvements help Laravel applications handle high traffic and complex workloads more effectively.
Real-Time Features and WebSocket Upgrades
Real-time web applications benefit from several improvements in Laravel 12’s event broadcasting and WebSocket support.
The WebSocket broadcasting mechanism has been optimized to reduce latency and improve connection handling. Events are now delivered more efficiently, even under heavy loads.
Integration between event broadcasting and the queue system has been tightened, ensuring that real-time updates are processed quickly and reliably.
Developer Experience and Debugging Improvements
Laravel 12 introduces several features to enhance the developer experience and simplify debugging.
# Unified Scaffolding Command
The new php artisan scaffold command allows developers to create a model, migration, controller, and factory with a single command:
Before (Laravel 11):
php
php artisan make:model Product php artisan make:controller ProductController php artisan make:migration create_products_table
After (Laravel 12):
php
php artisan scaffold Product
# AI-Powered Debugging Assistant
Laravel 12 also introduces an AI-powered debugging assistant. The new debug()->suggest() function analyzes variable output and provides actionable suggestions:
Before (Laravel 11):
php
dd($variable);
After (Laravel 12):
php
debug($variable)->suggest();
This helps developers diagnose issues faster by offering targeted suggestions based on common errors.
Additional Updates in Laravel 12
Laravel 12 includes several smaller updates that contribute to a more polished framework.
- The new frontend:install command replaces older UI scaffolding commands.
- Several older methods have been deprecated to improve framework consistency.
- Laravel 12 requires PHP 8.2 or higher to take advantage of modern PHP features and security updates.
Why Upgrade to Laravel 12?
Laravel 12 brings a host of improvements to security, performance, and developer experience. The new starter kits, enhanced query builder, improved API handling, and AI-powered debugging simplify Laravel development while making applications more scalable and secure.
For businesses, this translates to faster load times, more reliable authentication, and reduced development costs. The cleaner project structure and modern feature set make Laravel 12 an excellent choice for both new and existing projects.
Build with Laravel 12 – Contact Shiv Technolabs
If you’re looking to upgrade an existing Laravel app or start a new project, Shiv Technolabs can help.
Our experienced Laravel developers are well-versed in the latest updates and can implement them to maximize performance and scalability for your project.
Contact Shiv Technolabs today to discuss your Laravel development needs and see how Laravel 12 can transform your business.
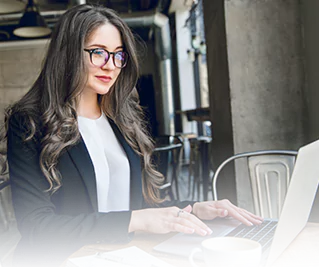