Augmented Reality (AR) and Virtual Reality (VR) are transforming the way users interact with digital content. AR overlays digital information onto the real world, enhancing user perception, while VR immerses users in a completely virtual environment. Both technologies offer unique ways to create engaging and interactive experiences.
Benefits of AR and VR in Mobile Applications
AR and VR provide new dimensions for mobile applications, allowing developers to create more immersive and interactive experiences. From gaming and entertainment to education and training, these technologies offer endless possibilities. AR can be used for navigation, retail, and real-time information display, while VR can create simulated environments for training, therapy, and entertainment.
- Improved User Interaction: AR and VR create engaging and interactive experiences.
- Better Learning Tools: Simulate real-world scenarios for training in various industries.
- Retail Engagement: Customers can try products virtually, reducing returns.
- Enhanced Gaming: Provides immersive and interactive environments.
- Real Estate Visualization: Virtual property tours save time and provide realistic views.
- Navigation Aid: Real-time directions overlaid on the real world.
- Tourism Enrichment: Adds interactive elements to historical sites and landmarks.
- Marketing Impact: Creates interactive ads and 3D product demonstrations.
- Remote Assistance: Offers detailed instructions overlaid on equipment.
- Social Media Fun: Adds virtual elements to photos and videos.
- Accessibility Improvement: Helps individuals with disabilities navigate and interact.
Market Trends and Use Cases for AR and VR
The market for AR and VR is rapidly growing, with significant investments and advancements in hardware and software. Use cases range from AR-based apps for interior design and navigation to VR-based training simulations and virtual tours. As technology advances, the adoption of AR and VR in various industries is expected to increase.
Setting Up the Development Environment
Setting up the development environment for Flutter app development services involves installing Flutter and configuring your system to support AR and VR functionalities. This includes setting up necessary plugins, ensuring compatibility with ARCore for Android and ARKit for iOS, and configuring emulators or physical devices for testing.
# Prerequisites for AR and VR Development
Before starting with AR and VR development in Flutter, you need a solid understanding of Flutter and Dart programming. Additionally, familiarity with Android and iOS development is beneficial. Ensure that your development environment is set up with the latest versions of Flutter, Dart, and the necessary AR/VR SDKs.
# Installing Flutter and Necessary Plugins
To begin, install Flutter and set up your development environment. Follow the official Flutter installation guide to set up Flutter on your operating system. Once Flutter is installed, you can start integrating AR and VR plugins into your Flutter project.
# Configuring the Android and iOS Emulators for AR/VR Testing
Testing AR and VR applications requires specific configurations for Android and iOS emulators. For AR applications, you need ARCore on Android devices and ARKit on iOS devices. Configure your emulators to support these technologies, or use real devices for testing.
Using ARCore and ARKit with Flutter
Integrating ARCore for Android and ARKit for iOS with Flutter involves setting up the necessary plugins and configuring your project to support augmented reality features. These powerful AR platforms allow Flutter developers to create immersive AR experiences by leveraging device capabilities.
# Introduction to ARCore and ARKit
ARCore and ARKit are Google’s and Apple’s AR development platforms, respectively. They provide the necessary tools to build AR applications by leveraging the device’s camera and sensors to create augmented experiences.
# Integrating ARCore in Flutter for Android
To integrate ARCore in a Flutter project, you need to add the ARCore plugin to your project. This involves setting up the Android project to include ARCore dependencies and configuring the “AndroidManifest.xml” file to support AR features.
1. Setting Up ARCore SDK
dependencies: arcore_flutter_plugin: ^0.0.9
2. Building a Simple AR Application
Create a simple AR application that detects surfaces and places 3D objects. Use the ARCore plugin to handle AR functionalities like surface detection and object placement.
# Integrating ARKit in Flutter for iOS
Similarly, integrating ARKit requires setting up the iOS project with ARKit dependencies and configuring the Info.plist file to enable AR capabilities.
1. Setting Up ARKit SDK
dependencies: arkit_plugin: ^0.6.0
2. Building a Simple AR Application
Develop an AR application that uses ARKit to detect surfaces and place 3D objects. Utilize the ARKit plugin to manage AR functionalities on iOS devices.
Flutter Plugins for AR and VR
Flutter offers a range of plugins to facilitate AR and VR development, such as “arcore_flutter_plugin” and “arkit_plugin” for AR, and “flutter_unity_widget” for VR. These plugins provide essential tools and functionalities to build interactive and immersive applications.
# Exploring Available Flutter AR/VR Plugins
There are several Flutter plugins available for AR and VR development. The “arcore_flutter_plugin” and “arkit_plugin” are popular choices for AR development on Android and iOS, respectively. For VR, plugins like “flutter_unity_widget” and “flutter_vr” can be used.
# Using the “arcore_flutter_plugin” for ARCore
The “arcore_flutter_plugin” simplifies the integration of ARCore in Flutter projects. It provides methods for surface detection, object placement, and interaction handling. By using this plugin, you can create robust AR applications for Android devices.
# Using the “arkit_plugin” for ARKit
The “arkit_plugin” offers a seamless way to integrate ARKit functionalities into Flutter projects. It supports surface detection, object placement, and AR interactions, enabling you to build feature-rich AR applications for iOS devices.
# Comparison of Features and Capabilities
While both plugins provide similar functionalities, there are differences in their capabilities and performance. ARCore is supported on a wide range of Android devices, while ARKit is exclusive to iOS devices. Evaluate the requirements of your project to choose the appropriate plugin.
Building an Augmented Reality App
Creating an augmented reality app in Flutter involves setting up the app structure, implementing AR features like object placement and surface detection, and handling user interactions. Using ARCore and ARKit plugins, developers can create engaging AR experiences on both Android and iOS.
# Creating a Basic AR App Structure
Start by setting up the basic structure of your AR application. Define the necessary widgets and layout elements to support AR functionalities. Use Flutter’s widget system to build a user interface that interacts with the AR environment.
# Implementing AR Features (e.g., Object Placement, Tracking)
Implement core AR features like object placement and tracking. Use the ARCore or ARKit plugin to detect surfaces and place 3D objects. Handle user interactions to allow manipulation of AR objects within the environment.
# Handling User Interactions in AR
User interactions are crucial for AR applications. Implement touch and gesture controls to allow users to interact with AR objects. Use Flutter’s gesture detection capabilities to handle these interactions seamlessly.
Building a Virtual Reality App
Developing a virtual reality app in Flutter requires setting up a VR environment, implementing features like 360-degree views and user navigation, and optimizing for performance. VR plugins enable developers to create immersive experiences that transport users to virtual worlds.
# Creating a Basic VR App Structure
For VR applications, set up the basic structure with the necessary widgets and layout elements. Define the VR environment and use Flutter’s widget system to create a user interface that immerses the user in a virtual world.
# Implementing VR Features (e.g., 360-Degree Views, Navigation)
Implement VR features such as 360-degree views and navigation within the virtual environment. Use VR plugins to manage the VR scene and handle user interactions. Ensure that the VR experience is smooth and responsive.
# Handling User Interactions in VR
User interactions in VR are different from AR. Implement controls for navigating the virtual environment, interacting with virtual objects, and handling user inputs. Use VR controllers or touch interactions to enhance the user experience.
Advanced Techniques in AR and VR Development
Advanced AR and VR development techniques include incorporating 3D models, adding spatial audio, and optimizing performance. These techniques enhance the realism and interactivity of AR/VR applications, providing users with more immersive experiences.
# Enhancing AR Experiences with 3D Models
Incorporate 3D models to create more realistic and engaging AR experiences. Use 3D modeling tools to create custom objects and import them into your Flutter project. Optimize models for performance to ensure smooth rendering.
# Improving VR Experiences with Spatial Audio
Spatial audio adds an extra layer of immersion to VR applications. Implement spatial audio to simulate realistic sound environments. Use audio libraries and plugins to manage audio sources and create dynamic soundscapes.
# Performance Optimization for AR/VR Applications
AR and VR applications can be resource-intensive. Optimize performance by managing resources efficiently, reducing latency, and ensuring smooth rendering. Use profiling tools to identify and address performance bottlenecks.
Testing and Debugging AR and VR Apps
Testing AR and VR apps on real devices is crucial for ensuring a smooth user experience, as emulators may not fully support AR/VR features. Debugging tools and techniques help identify and fix issues related to performance, user interactions, and device compatibility.
# Testing AR Applications on Real Devices
Testing on real devices is essential for AR applications. Emulators may not fully support AR features, so use physical devices to test and debug your AR applications. Pay attention to device-specific issues and optimize accordingly.
# Testing VR Applications on Real Devices
Similarly, test VR applications on real devices to ensure a seamless user experience. Use VR headsets and controllers to test interactions and navigation within the virtual environment. Address any device-specific challenges that arise.
# Debugging Common Issues in AR/VR Development
Debugging AR and VR applications can be challenging. Use Flutter’s debugging tools to identify and fix issues. Common problems include sensor inaccuracies, performance bottlenecks, and user interaction glitches. Address these issues systematically to improve the overall experience.
Deploying AR and VR Apps
Deploying AR and VR apps involves preparing them for release by optimizing performance, following platform-specific guidelines, and submitting them to app stores. Thorough testing and quality assurance are essential to meet the standards of Google Play Store and Apple App Store.
# Preparing Your AR/VR App for Production
Before releasing your AR/VR app, ensure it is thoroughly tested and optimized. Prepare the app for production by following platform-specific guidelines and best practices. Optimize performance, handle edge cases, and ensure a smooth user experience.
# Publishing AR Apps on Google Play Store
Publish your AR app on the Google Play Store by following the submission guidelines. Prepare the necessary assets, such as app icons, screenshots, and descriptions. Test the app thoroughly to meet the store’s quality standards.
# Publishing VR Apps on Apple App Store
For VR apps, follow the Apple App Store guidelines for submission. Prepare the required assets and ensure the app meets the store’s quality standards. Test on multiple devices to ensure compatibility and performance.
# Marketing and User Acquisition Strategies for AR/VR Apps
Promote your AR/VR app through various marketing channels. Use social media, app store optimization (ASO), and targeted advertising to reach your audience. Collect user feedback to improve the app and drive user engagement.
Future of AR and VR in Flutter
The future of AR and VR in Flutter looks promising, with continuous advancements in technology and increasing support for AR/VR features. As the Flutter ecosystem grows, developers can expect more robust tools and resources for creating cutting-edge AR and VR applications.
# Emerging Trends and Technologies in AR/VR
Stay updated with the latest trends and technologies in AR and VR. Advances in hardware, software, and development tools continue to push the boundaries of what is possible in AR/VR applications.
# Potential Challenges and Solutions
AR and VR development come with unique challenges, such as performance optimization, device compatibility, and user interaction design. Address these challenges by staying informed and adapting to new technologies and best practices.
# The Role of Flutter in the Future of AR/VR Development
Flutter’s flexibility and performance make it a strong contender for AR and VR development. As the Flutter ecosystem grows, expect more robust support for AR and VR features, enabling developers to create cutting-edge applications.
Conclusion
This guide has covered the essential concepts and techniques for integrating AR and VR in Flutter apps. From setting up the development environment to implementing advanced features, you now have a roadmap to create immersive AR and VR experiences.
Follow best practices for AR and VR development to ensure a smooth and engaging user experience. Optimize performance, test on real devices, and stay updated with the latest trends and tools.
Take your AR and VR development projects to the next level with our Flutter app development services in USA. Our expert team stays informed about the latest advancements in AR and VR technologies to deliver cutting-edge solutions for your needs.
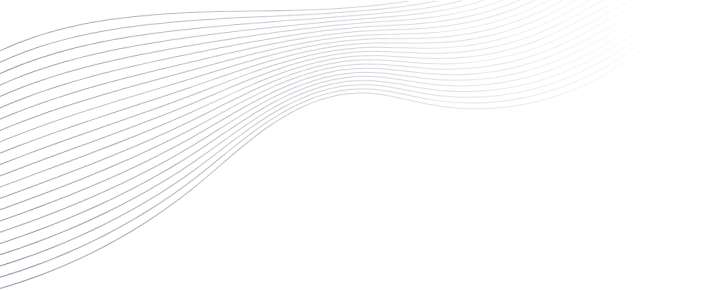
Revolutionize Your Digital Presence with Our Mobile & Web Development Service. Trusted Expertise, Innovation, and Success Guaranteed.