Table of Contents
Building scalable and efficient web applications is crucial for businesses today. Combining Next.js with Docker provides a practical and powerful approach for developers who want to create applications that can handle growth and performance challenges with ease. In this guide, we’ll explore how to integrate Next.js with Docker, including writing a Next.js 14 Dockerfile, handling Next.js Docker environment variables, and providing insights for Next.js developers and companies looking for Next.js development services.
What is Next.js?
Next.js is a popular React framework that offers server-side rendering (SSR), static site generation (SSG), and API routing features. These characteristics make it an excellent choice for modern web development, helping developers deliver applications that are fast, dynamic, and efficient.
Why Use Docker with Next.js?
Docker allows developers to package applications and their dependencies into containers. By running Next.js inside Docker, developers can standardize their environment, reducing issues related to differences in setup between development, testing, and production stages. This approach enhances the collaboration process and makes it easier to scale applications as they grow.
Also Read:- How Do You Set Up Flash Messages in Node.js with connect-flash?
# Setting Up Next.js with Docker
Let’s walk through the steps to containerize a Next.js application using Docker.
Step 1: Install Docker
Before you begin, Docker should be installed on your machine. You can download Docker from the official Docker website, then verify the installation by running the following command:
docker --version
Step 2: Create a Next.js Project
If you don’t have a Next.js project, you can create one with this command:
npx create-next-app@latest
This will generate a new Next.js project in your chosen directory.
Step 3: Creating a Dockerfile for Next.js
The Dockerfile defines the instructions needed to build a Docker image for your application. Below is an example Next.js 14 Dockerfile:
# Use an official Node.js runtime as a parent image FROM node:16-alpine AS base # Set the working directory inside the container WORKDIR /app # Copy package.json and package-lock.json files COPY package*.json ./ # Install dependencies RUN npm install # Copy the rest of the app's code to the container COPY . . # Build the Next.js app RUN npm run build # Set the environment variable to run the Next.js app ENV NODE_ENV=production # Expose the port the app runs on EXPOSE 3000 # Start the app CMD ["npm", "start"]
This Dockerfile builds your Next.js application and prepares it for production use.
Managing Environment Variables in Docker
Handling environment variables is important when working with Docker and Next.js. These variables allow your application to work in different environments by adjusting settings without changing the code.
To manage Next.js Docker environment variables, create a .env file in the project root directory. Here’s an example:
# Environment variables for Docker NEXT_PUBLIC_API_URL=https://api.example.com NODE_ENV=production
In the Dockerfile, you can copy the .env file into the container to make these variables available:
# Copy .env file COPY .env .env
Alternatively, you can pass the environment variables during the container run:
docker run -p 3000:3000 --env-file .env my-nextjs-app
This makes sure your app can adjust according to the environment it is running in, without needing to modify the code.
Benefits for Next.js Developers Using Docker
Developers using Next.js in combination with Docker enjoy several advantages:
- Consistent Environment: Docker provides a stable environment, ensuring that the app behaves the same on different machines or servers. This reduces debugging time related to differences in setups across development, testing, and production environments.
- Improved Collaboration: Teams of Next.js developers can easily share their work, as Docker ensures that everyone uses the same environment. This minimizes configuration issues and helps developers focus on writing code.
- Scalability: Docker makes it easier to manage and scale Next.js applications. Running multiple containers for different parts of the application can handle larger user bases and increased traffic.
- Simplified Testing and Debugging: By isolating the environment, Docker allows developers to test features and find issues more easily, without affecting the overall system.
# Using Docker Compose with Next.js
For more complex setups, where you need to run multiple services (like a database), Docker Compose simplifies managing different containers. It allows you to define all services in a single file.
Here’s an example docker-compose.yml for a Next.js application:
version: '3' services: app: image: my-nextjs-app build: context: . dockerfile: Dockerfile ports: - "3000:3000" environment: - NODE_ENV=development
To start your containers, run:
docker-compose up
This command will build and run your Next.js application, simplifying the management of multiple services.
Optimizing Docker Images for Next.js
To keep your Next.js Docker image lightweight and efficient, use multi-stage builds. This method allows you to separate the building phase from the production phase, keeping the final image size smaller.
Here’s an example:
# Build stage FROM node:16-alpine AS build WORKDIR /app COPY package*.json ./ RUN npm install COPY . . RUN npm run build # Production stage FROM node:16-alpine AS production WORKDIR /app COPY --from=build /app ./ EXPOSE 3000 CMD ["npm", "start"]
This setup reduces the final image size, which leads to faster builds and reduced resource usage.
Next.js Development Services and Docker Integration
Many companies today seek Next.js development services to create high-performing, scalable applications. These services often include Docker integration, which helps to streamline development workflows and improve collaboration among team members.
By using Docker, Next.js developers can focus on building quality applications without worrying about differences in local environments. Whether your business is looking to start a new project or scale an existing one, finding the right Next.js development services that incorporate Docker can significantly improve project outcomes.
Conclusion
Integrating Next.js with Docker is an excellent way to build scalable and efficient web applications. Docker allows developers to run Next.js in isolated environments, ensuring that the application behaves consistently across different stages of the development process. From setting up a Next.js 14 Dockerfile to managing Next.js Docker environment variables, the process becomes much easier with Docker.
By using Docker, Next.js developers and businesses looking for Next.js development services? Shiv Technolab’s skilled developers can build scalable and efficient applications, ensuring that they are ready for future growth and performance demands. Docker not only simplifies development but also improves the overall reliability and scalability of web applications.
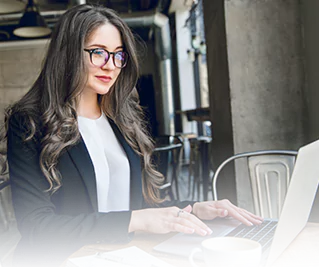