In the fast-paced world of business, exchanging contact information efficiently and effectively is crucial. Traditional business cards, while still widely used, often lead to a clutter of paper and the risk of losing important contacts. A React Native Business Card Scanner app can revolutionize the way business professionals handle contact information. This detailed guide will walk you through the process of creating such an app, highlighting key features, technologies involved, and best practices for development.
Overview of a Business Card Scanner App
A business card scanner app aims to digitize the information on physical business cards, storing it in a structured and accessible format on a mobile device. Users can quickly scan cards, save contact details, and retrieve them as needed, all from the convenience of their smartphones. React Native, a popular framework for building cross-platform mobile applications, provides an ideal platform for developing this type of app.
Key Features of the App
# OCR (Optical Character Recognition) Integration:
The core functionality of a business card scanner app is the ability to recognize text from images. This is achieved through OCR technology. Libraries like Tesseract or Google Vision can be integrated into the React Native app to extract text from scanned images. An OCR scanner for business cards is crucial for accurately capturing contact details.
# Contact Management:
Users should be able to store scanned contacts, categorize them, and access them easily. This involves creating a user-friendly interface for viewing, editing, and organizing contacts.
# Synchronization:
To avoid losing data, the app should offer synchronization with cloud services. This allows users to back up their contacts and access them from multiple devices.
# User Authentication:
Secure user authentication restricts access to authorized users. Implementing OAuth or integrating with popular services like Google or Facebook can streamline this process.
# Export and Share:
Users may want to export their contact list to other applications like email clients or CRM systems. Providing options to export contacts in various formats (e.g., CSV, VCF) enhances the app’s utility.
# Search and Filter:
As the number of stored contacts grows, users need efficient ways to search and filter through their database. Implementing robust search functionality is essential for a smooth user experience.
Setting Up the Development Environment
# Install React Native:
To get started with React Native, you need to install Node.js and the React Native CLI. Follow the official React Native documentation for the latest installation instructions.
# Set Up a New Project:
Use the React Native CLI to create a new project. For instance:
npx react-native init BusinessCardScanner
# Install Required Libraries:
Install necessary libraries for OCR, camera access, and other functionalities. Examples include:
npm install react-native-camera npm install tesseract.js
# Link Native Modules:
Some libraries require linking native modules. This can usually be done with:
npx react-native link react-native-camera
Implementing Core Functionalities
# Camera Integration:
Use the “react-native-camera” library to integrate camera functionality. This allows users to capture images of business cards directly within the app. This makes it a powerful document and business card scanner, providing a smooth user experience.
import { RNCamera } from 'react-native-camera'; const CameraScreen = () => { return ( <RNCamera style={{ flex: 1 }} type={RNCamera.Constants.Type.back} captureAudio={false} > {/* Camera UI */} </RNCamera> ); };
# Text Recognition:
Integrate an OCR library like Tesseract.js to recognize and extract text from the captured images. An effective OCR scanner for business cards ensures that all details are accurately captured and stored.
import Tesseract from 'tesseract.js'; const recognizeText = async (imagePath) => { const result = await Tesseract.recognize(imagePath, 'eng'); return result.data.text; };
# Contact Storage:
Use a local database like SQLite or AsyncStorage to store contact details. For more advanced features, consider using Realm or integrating with a cloud database like Firebase.
import AsyncStorage from '@react-native-async-storage/async-storage'; const saveContact = async (contact) => { const existingContacts = await AsyncStorage.getItem('contacts'); const contacts = existingContacts ? JSON.parse(existingContacts) : []; contacts.push(contact); await AsyncStorage.setItem('contacts', JSON.stringify(contacts)); };
# User Authentication:
Implement authentication using Firebase Authentication or a similar service to manage user sessions securely.
import auth from '@react-native-firebase/auth'; const signInWithGoogle = async () => { // Sign in logic };
# Synchronization:
Implement synchronization with cloud storage solutions to allow users to back up and access their contacts from any device.
import firestore from '@react-native-firebase/firestore'; const syncContacts = async (userId, contacts) => { const userContactsRef = firestore().collection('users').doc(userId).collection('contacts'); contacts.forEach(async (contact) => { await userContactsRef.add(contact); }); };
Best Practices for Development
# User Experience:
Focus on creating a smooth and intuitive user interface. Use React Native’s component library and styling options to design a clean and responsive app.
# Performance Optimization:
Optimize image processing and text recognition to ensure the app performs well on various devices. This might include resizing images before processing and running OCR tasks in background threads.
# Security:
Keep user data stored securely, both locally and in the cloud. Implement encryption for sensitive data and follow best practices for authentication.
# Testing:
Thoroughly test the app on both Android and iOS platforms to identify and fix any issues. Use tools like Jest for unit testing and Detox for end-to-end testing.
# Accessibility:
Ensure that the app is accessible to all users by following accessibility guidelines. This includes providing alternative text for images, ensuring proper color contrast, and supporting screen readers.
Detailed Implementation Steps
# Setting Up Navigation:
Use React Navigation to set up the app’s navigation structure. This might include a stack navigator for managing different screens, such as the camera screen, contact list, and settings.
import { NavigationContainer } from '@react-navigation/native'; import { createStackNavigator } from '@react-navigation/stack'; const Stack = createStackNavigator(); const App = () => { return ( <NavigationContainer> <Stack.Navigator initialRouteName="Home"> <Stack.Screen name="Home" component={HomeScreen} /> <Stack.Screen name="Camera" component={CameraScreen} /> <Stack.Screen name="Contacts" component={ContactsScreen} /> </Stack.Navigator> </NavigationContainer> ); };
# Camera Screen UI:
Design the camera screen UI to include a button for capturing images and a preview area for displaying the captured image. This setup is vital for a React Native ID Card Scanner as well as for business cards.
import React, { useRef } from 'react'; import { View, Button } from 'react-native'; import { RNCamera } from 'react-native-camera'; const CameraScreen = ({ navigation }) => { const cameraRef = useRef(null); const takePicture = async () => { if (cameraRef.current) { const options = { quality: 0.5, base64: true }; const data = await cameraRef.current.takePictureAsync(options); navigation.navigate('Preview', { imageUri: data.uri }); } }; return ( <View style={{ flex: 1 }}> <RNCamera ref={cameraRef} style={{ flex: 1 }} type={RNCamera.Constants.Type.back} captureAudio={false} /> <Button title="Capture" onPress={takePicture} /> </View> ); };
# Text Recognition Processing:
After capturing an image, process it using Tesseract.js to recognize text. Display the extracted text in a preview screen where users can edit or save the contact details.
import React, { useState, useEffect } from 'react'; import { View, Text, Image, Button } from 'react-native'; import Tesseract from 'tesseract.js'; const PreviewScreen = ({ route, navigation }) => { const { imageUri } = route.params; const [recognizedText, setRecognizedText] = useState(''); useEffect(() => { const recognizeText = async () => { const result = await Tesseract.recognize(imageUri, 'eng'); setRecognizedText(result.data.text); }; recognizeText(); }, [imageUri]); return ( <View style={{ flex: 1 }}> <Image source={{ uri: imageUri }} style={{ flex: 1 }} /> <Text>{recognizedText}</Text> <Button title="Save" onPress={() => navigation.navigate('SaveContact', { text: recognizedText })} /> </View> ); };
# Saving and Managing Contacts:
Create a screen for saving new contacts and managing existing ones. Use AsyncStorage to persist contact data locally.
import React, { useState } from 'react'; import { View, TextInput, Button } from 'react-native'; import AsyncStorage from '@react-native-async-storage/async-storage'; const SaveContactScreen = ({ route, navigation }) => { const { text } = route.params; const [name, setName] = useState(''); const [phone, setPhone] = useState(''); const saveContact = async () => { const contact = { name, phone, details: text }; const existingContacts = await AsyncStorage.getItem('contacts'); const contacts = existingContacts ? JSON.parse(existingContacts) : []; contacts.push(contact); await AsyncStorage.setItem('contacts', JSON.stringify(contacts)); navigation.navigate('Contacts'); }; return ( <View> <TextInput placeholder="Name" value={name} onChangeText={setName} /> <TextInput placeholder="Phone" value={phone} onChangeText={setPhone} /> <Button title="Save Contact" onPress={saveContact} /> </View> ); };
# Viewing and Searching Contacts:
Implement a screen for viewing and searching through saved contacts. Provide options to edit or delete contacts as needed.
import React, { useState, useEffect } from 'react'; import { View, Text, FlatList, TextInput, Button } from 'react-native'; import AsyncStorage from '@react-native-async-storage/async-storage'; const ContactsScreen = ({ navigation }) => { const [contacts, setContacts] = useState([]); const [searchQuery, setSearchQuery] = useState(''); useEffect(() => { const loadContacts = async () => { const storedContacts = await AsyncStorage.getItem('contacts'); if (storedContacts) { setContacts(JSON.parse(storedContacts)); } }; loadContacts(); }, []); const filteredContacts = contacts.filter(contact => contact.name.toLowerCase().includes(searchQuery.toLowerCase()) ); return ( <View> <TextInput placeholder="Search Contacts" value={searchQuery} onChangeText={setSearchQuery} /> <FlatList data={filteredContacts} keyExtractor={(item, index) => index.toString()} renderItem={({ item }) => ( <View> <Text>{item.name}</Text> <Text>{item.phone}</Text> <Text>{item.details}</Text> <Button title="Edit" onPress={() => navigation.navigate('EditContact', { contact: item })} /> <Button title="Delete" onPress={async () => { const newContacts = contacts.filter(c => c !== item); setContacts(newContacts); await AsyncStorage.setItem('contacts', JSON.stringify(newContacts)); }} /> </View> )} /> </View> ); };
Conclusion
Building a React Native Business Card Scanner app involves integrating several technologies and focusing on creating a user-friendly experience. By using OCR technology, secure storage solutions, and robust authentication methods, you can create an app that transforms how professionals manage their contacts. This guide provides a thorough overview of the steps involved, from setting up the development environment to implementing core features and best practices for a successful app.
With careful planning and execution, your React Native Business Card Scanner app can become an essential tool for business professionals, making it easier for them to manage and organize their contacts efficiently.
In addition to being a powerful document and business card scanner, this application can also serve as a React Native ID Card Scanner. By adapting the same OCR technology, you can create an app that reads and stores ID card information, making it versatile for various professional uses.
Furthermore, a Visiting Cards Reader React Native app can greatly enhance the efficiency of managing contact details. By incorporating the best practices outlined in this guide, developers can create an intuitive, reliable, and highly functional application for all contact management needs. For those looking to build such a solution, partnering with a React Native development company in Turkey can provide the expertise and local insights necessary to deliver a high-quality app.
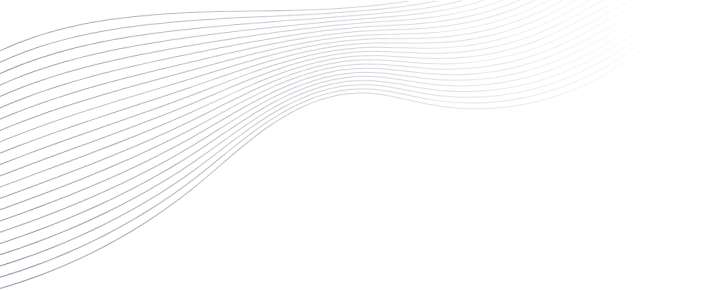
Revolutionize Your Digital Presence with Our Mobile & Web Development Service. Trusted Expertise, Innovation, and Success Guaranteed.