Table of Contents
In today’s digital world, the speed and efficiency of web applications are crucial. Users expect quick responses and seamless experiences. Django, a high-level Python web framework, is designed to help developers create web applications rapidly. However, to get the best performance from Django, it’s essential to apply various optimization techniques. This guide will cover twelve key tips to improve Django’s performance, ensuring your web applications run smoothly and efficiently.
Recent studies indicate that slow-loading websites result in a 25% increase in bounce rates. In contrast, optimizing web applications for speed can lead to a 20% increase in user engagement and satisfaction. With over 50% of users expecting a webpage to load in less than two seconds, ensuring your Django application performs well is more important than ever. Python development services play a crucial role in achieving these performance benchmarks, making it vital to implement best practices for speed and efficiency.
Moreover, a report by Google reveals that as page load time goes from one second to five seconds, the probability of a user bouncing increases by 90%. Additionally, businesses with faster websites are shown to have a higher conversion rate, with a 1-second delay in page response potentially leading to a 7% reduction in conversions. These statistics highlight the importance of optimizing Django applications not only for user experience but also for business success and profitability.
How are Django and Python Related?
Django is built using Python, a programming language known for its simplicity and readability. This relationship brings several benefits to web development:
- Ease of Learning and Use: Python’s simple and readable syntax makes it accessible for beginners and efficient for experienced developers. Django inherits this ease of use, allowing developers to quickly build and maintain web applications.
- Rich Ecosystem: Python has a vast collection of libraries and frameworks, which Django can leverage to extend its capabilities. This means developers have access to a wide range of tools for various tasks, from data manipulation to machine learning.
- Strong Community Support: Both Python and Django have active communities. This support network provides abundant resources, tutorials, and plugins, helping developers find solutions to problems and share best practices.
- Performance and Efficiency: Python’s performance features, such as its dynamic typing and memory management, combined with Django’s efficient framework, result in fast and responsive web applications.
Django Framework
Django is a high-level framework that encourages rapid development and clean, pragmatic design. It follows the Model-Template-View (MTV) architecture, which helps developers organize their code effectively. Here are some key features of the Django framework:
# MTV Architecture:
- Model: Manages the database schema and operations, making it easier to handle data.
- Template: Handles the presentation layer, allowing developers to define the structure of the HTML pages.
- View: Contains the business logic and interacts with both the model and the template to process requests and return responses.
# DRY Principle:
Django emphasizes the “Don’t Repeat Yourself” principle, which promotes code reuse and reduces redundancy. This makes the codebase easier to maintain and update.
# Security Features:
Django comes with built-in protections against common web threats like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). These features help developers create secure applications without needing to implement these protections from scratch.
# Scalability:
Django is designed to handle applications of any size, from small projects to large-scale, high-traffic websites. Its architecture allows for efficient scaling as the application’s user base grows.
# Admin Interface:
Django includes a powerful admin interface that simplifies the management of application data. This interface allows developers to quickly perform administrative tasks without writing additional code.
# ORM (Object-Relational Mapping):
Django’s ORM lets developers interact with the database using Python code instead of SQL queries. This abstraction layer makes database operations simpler and more intuitive.
# Extensible and Modular:
Django’s modular design means developers can add or remove components as needed. This flexibility allows for customization to fit specific project requirements.
Also read : The Ultimate Guide to Python and Machine Learning in Cybersecurity
Tips to Optimize Django Performance
Improving the performance of your Django application is crucial for providing a fast and responsive user experience. By focusing on key areas such as database management, code efficiency, and caching, you can significantly boost your application’s speed and reliability. Here are twelve essential tips to help you achieve optimal performance with your Django projects.
1) Database Optimization
Databases are often the backbone of web applications, and their performance directly impacts the overall speed of your Django app. Here are some ways to improve database performance:
- Use Indexes: Proper indexing of your database tables can speed up query performance significantly. Ensure that the fields frequently used in queries are indexed. This minimizes the time taken to search through the database and retrieve the required data.
- Avoid N+1 Query Problem: This issue arises when your code makes separate database queries for each object in a collection. Using select_related and prefetch_related can help fetch related objects in fewer queries. This reduces the number of database hits and improves response times.
- Optimize Database Schema: Regularly review and update your database schema. Remove unnecessary fields and tables to keep the database lean. Streamlining your schema can lead to faster query performance and reduced load times.
2) Code Optimization
Writing efficient code is fundamental to achieving good performance. Here are some tips for optimizing your Django code:
- Avoid Redundant Code: Keep your codebase clean by removing duplicate or unnecessary code. This makes the application more efficient and easier to maintain. Cleaner code leads to fewer processing steps and faster execution.
- Use Efficient Data Structures: Choose the right data structures that provide optimal performance for the tasks at hand. For example, use dictionaries for fast lookups instead of lists. The right data structure can significantly reduce the time complexity of operations.
- Limit the Use of Loops: Minimize the use of nested loops and consider alternatives that achieve the same result with fewer iterations. This helps in reducing the processing time, especially for large datasets.
3) Enable Caching
Caching is a powerful technique that stores copies of files or data in a temporary storage area to reduce load times. Implement caching to improve the performance of your Django application:
- Database Query Caching: Cache the results of expensive database queries to reduce load times on subsequent requests. This minimizes the need to repeatedly execute complex queries.
- Template Fragment Caching: Cache parts of templates that do not change often to speed up rendering times. This reduces the need to regenerate the same content repeatedly.
- Full Page Caching: Cache entire pages to serve them quickly without the need for processing the request each time. This is especially useful for pages that do not change frequently.
4) Concept of Laziness
Lazy loading is a technique that delays the loading of objects until they are needed, which can save resources and improve performance:
- Deferred Loading: Only load objects when they are accessed, rather than loading everything at once. This can significantly reduce the initial load time of your application by fetching data only when it is actually needed.
- QuerySet Lazy Loading: In Django, QuerySets are lazy by default. This means they do not hit the database until the data is actually needed, which helps in managing database load more effectively. This approach ensures that unnecessary database operations are avoided.
5) Caching: A Powerful Technique
Caching can dramatically improve the performance of your Django application by reducing the time it takes to serve content:
- Memcached and Redis: Use these caching systems to store and retrieve frequently accessed data quickly. They are designed for high-performance scenarios and can handle large amounts of data efficiently. Memcached and Redis are particularly useful for reducing database load.
- File-Based Caching: For smaller projects, file-based caching can be a simple and effective solution to speed up your application. This method involves storing cached data in the file system.
6) Using Performance Booster Functions
Certain functions and libraries are specifically designed to improve performance in Django applications:
- Django Debug Toolbar: This tool helps identify performance bottlenecks by providing detailed information about each request and the time it takes to process them. It is invaluable for spotting and addressing inefficiencies.
- Profiling Tools: Use profiling tools to analyze the performance of your code and identify areas that need improvement. These tools provide insights into how different parts of your application are performing and where optimizations are needed.
7) Laziness
Django’s built-in lazy loading capabilities can be further optimized to improve performance:
- Lazy Evaluation of Variables: Use lazy evaluation to delay the computation of values until they are actually needed. This can save processing time and resources, especially for computations that may not always be necessary.
- Lazy Loading of Media Files: Load images and other media files only when they are required, reducing initial page load times. This technique is particularly beneficial for pages with a lot of media content.
8) Configuration
Proper configuration of your Django settings can have a significant impact on performance:
- Database Connection Pooling: Use connection pooling to manage database connections more efficiently and reduce latency. This technique involves maintaining a pool of open database connections that can be reused, which helps in improving the performance and responsiveness of your application.
- Static and Media File Handling: Configure Django to serve static and media files efficiently, either through a dedicated server or a content delivery network (CDN). This offloads the serving of static content from your main server, reducing load and improving the overall performance of your application.
9) Specific Functions
Certain Django functions can be used to enhance performance in specific scenarios:
- Bulk Create and Update: Use bulk operations to insert or update multiple records in the database in a single query, which is faster than doing it one by one. This can significantly reduce the number of database transactions and improve performance.
- QuerySet Methods: Methods like only, defer, and values can help in reducing the amount of data fetched from the database, improving query performance. These methods allow you to retrieve only the data you need, minimizing overhead and speeding up queries.
10) Upgradation Using Newer Version
Keeping your Django version up to date ensures that you benefit from the latest performance improvements and security patches:
- Regular Updates: Regularly update Django and its dependencies to the latest versions to take advantage of new features and optimizations. Staying current with updates can bring performance enhancements and bug fixes, helping to keep your application secure and efficient.
- Deprecation Warnings: Pay attention to deprecation warnings and update your code accordingly to avoid potential performance issues in future releases. Addressing these warnings ensures that your application remains compatible with future updates and minimizes the risk of encountering deprecated features.
11) Use Performance Booster to Handle Big Number of Requests
Handling a large number of requests efficiently is critical for high-traffic applications:
- Load Balancing: Use load balancers to distribute incoming requests across multiple servers, ensuring that no single server becomes a bottleneck. This helps in managing traffic and improving response times, leading to a more reliable and scalable application.
- Asynchronous Processing: Implement asynchronous processing for tasks that can be performed in the background, freeing up resources for handling incoming requests. This approach is useful for tasks that do not require immediate responses, such as sending emails or processing large datasets, and helps in maintaining smooth application performance.
12) HTTP Performance
Optimizing HTTP performance can lead to faster page load times and a better user experience:
- Keep-Alive Connections: Enable keep-alive connections to reduce the overhead of establishing new connections for each request. This keeps the connection open for multiple requests, reducing latency and improving overall response times.
- Compression: Use compression techniques like Gzip to reduce the size of the data transferred over the network, speeding up load times. This is particularly effective for text-based content such as HTML, CSS, and JavaScript files, leading to faster page loading and reduced bandwidth usage.
Also read : Node.js or Python: Which Backend Technology is Best for Your Website?
Why it is Important to Optimize Django Queries Correctly?
Optimizing Django queries is crucial for the overall performance of your web application. Efficient queries can drastically reduce load times, improve user experience, and decrease server load, making your application faster and more scalable. Here are several reasons why focusing on query optimization is essential:
# Speed and Responsiveness
Efficient queries mean faster data retrieval. When your application can quickly fetch and display data, it significantly improves the user experience. Slow queries, on the other hand, can cause noticeable delays, leading to frustration and potentially driving users away.
# Reduced Server Load
Optimized queries reduce the strain on your database server. When your queries are efficient, they consume fewer resources, such as CPU and memory. This allows your server to handle more requests simultaneously, improving the overall scalability of your application.
# Cost Efficiency
Reducing the load on your server can also lead to cost savings. Optimized queries mean your application can run efficiently on less powerful hardware or lower cloud service tiers, reducing your infrastructure costs. Additionally, efficient queries can reduce the need for extensive database scaling, which can be costly.
# Better User Experience
Users expect quick responses when interacting with web applications. By optimizing your queries, you ensure that your application meets these expectations. Fast loading times and smooth interactions enhance user satisfaction and encourage them to spend more time on your site or app.
# Prevention of Bottlenecks
Poorly optimized queries can become bottlenecks, slowing down the entire application. Identifying and addressing these bottlenecks ensures that one slow query does not impact the performance of other parts of your application. This is particularly important as your application grows and handles more data and users.
# Improved Application Reliability
Efficient queries contribute to the stability and reliability of your application. They reduce the likelihood of timeouts and errors that can occur when the database is overloaded. A reliable application builds user trust and reduces the frequency of performance-related issues.
# Scalability
As your application grows, the amount of data it processes will increase. Efficient queries are essential for scalability, allowing your application to handle larger datasets and higher user volumes without a corresponding drop in performance. This scalability is crucial for the long-term success of your application.
# Enhanced Security
Efficient queries often mean fewer data points are exposed and processed at any given time. This can enhance security by limiting the amount of data handled in each transaction, reducing the risk of data breaches and making it easier to implement effective security measures.
Conclusion
Improving Django performance involves focusing on various critical areas such as database management, code efficiency, caching, and configuration. Each aspect plays an essential role in making sure your Django applications run swiftly and reliably, providing users with a positive and responsive experience. By concentrating on efficient queries, reducing server load, and following best coding practices, you can significantly enhance your application’s speed and dependability. A well-optimized application not only boosts user satisfaction but also contributes to cost savings and scalability, laying the groundwork for future growth and success.
If you’re aiming to build high-performance web applications, Shiv Technolabs is here to assist you. As a premier Python Development company in Saudi Arabia, we specialize in creating scalable and efficient Django applications that meet your business requirements. Our skilled team of developers is dedicated to delivering top-quality solutions that ensure your web applications perform at their best. Partner with Shiv Technolabs to benefit from our extensive expertise and exceptional service in Python and Django development. Reach out to us today to elevate your web applications to new heights.
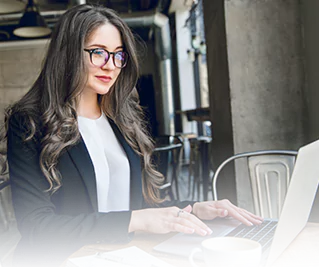