Table of Contents
In the modern era of app development, APIs (Application Programming Interfaces) play a crucial role in enabling applications to interact with each other seamlessly. APIs serve as the bridge between different software systems, enabling them to share data and functionality. However, when it comes to designing an efficient system, the debate often revolves around server-side APIs and how they differ from client-side APIs. This article explores server-side APIs, their importance, and their role in shaping dynamic and robust applications, especially for mobile app frameworks like React Native.
What Are Server-Side APIs?
Server-side APIs are a type of interface that resides on the server, acting as a middle layer between the client (such as a mobile app or web browser) and the server where the data resides. These APIs process requests from the client, fetch or modify the required data from the database, and then return the processed response back to the client.
In simpler terms, server-side APIs are responsible for:
- Managing requests from the front end (user interface).
- Querying databases or external services to fetch data.
- Applying business logic to process the data.
- Sending structured responses back to the client.
For example, in a React Native app for eCommerce, the client (app) sends a request to the server-side API to fetch product details. The API retrieves the data from the database, processes it, and sends the information back to the app, where it is displayed to the user.
Why Server-Side APIs Are Crucial for Modern Applications?
Server-side APIs are indispensable for modern applications because they ensure efficient communication between different parts of a software ecosystem. Here’s why they are essential:
# Data Security and Privacy
Server-side APIs keep sensitive operations, such as authentication, data validation, and database interactions, secure by keeping them on the server. Unlike client-side APIs, which can be exposed to users and malicious actors, server-side APIs are protected by server-level security protocols.
# Efficient Data Processing
Server-side APIs allow complex data processing and business logic to be handled on the server, reducing the load on the client device. This is particularly important for mobile apps where hardware resources are limited.
# Scalability
Server-side APIs are built to handle high volumes of requests and can be scaled as the user base grows. They work efficiently with server clusters and load balancers to distribute workloads, ensuring smooth performance even during peak traffic.
# Cross-Platform Support
Server-side APIs provide a unified backend that can serve multiple platforms, including mobile apps, web apps, and IoT devices. This simplifies development and maintenance by reducing redundant work across platforms.
# Real-Time Updates
Server-side APIs facilitate real-time updates, such as notifications, chat messages, or live data feeds, by leveraging technologies like WebSockets or Server-Sent Events (SSE). This enhances the responsiveness of applications, providing users with a dynamic experience.
Server-Side vs Client-Side APIs
APIs can operate either on the server side or the client side, depending on their intended function and location in the system architecture. Both approaches serve distinct purposes, and understanding the difference is essential for building efficient applications. Below is an in-depth analysis of server-side and client-side APIs, focusing on how they work, their strengths, and weaknesses.
# Overview
- Server-Side APIs: These are hosted on the server and act as intermediaries between the client (such as a mobile or web app) and the backend systems (databases, third-party services, etc.). They process requests from clients, perform server-side operations (like querying databases), and return structured responses.
- Client-Side APIs: These APIs operate directly on the client (user’s device/browser). They enable client-side applications to perform specific actions, such as rendering UI components or fetching public-facing data.
# Core Differences
Aspect | Server-Side APIs | Client-Side APIs |
---|---|---|
Location | Resides on the server. | Resides on the client (e.g., web browser or mobile app). |
Accessibility | Accessed by client applications via HTTP/HTTPS calls. | Executes directly on the user’s device/browser. |
Security | More secure since the logic and data are handled server-side. | Less secure; API keys and logic can be exposed in the client code. |
Data Processing | Handles complex operations like database queries and business logic. | Limited to lightweight tasks, such as rendering UI elements. |
Performance | Optimized for handling heavy computations and high-volume requests. | Dependent on the client device’s processing capabilities. |
Latency | May introduce additional latency due to server communication. | Low latency as it operates directly on the device. |
Offline Functionality | Limited offline functionality unless caching mechanisms are in place. | Can provide offline support if data is preloaded or cached locally. |
Server-side APIs are typically the preferred choice for applications that require robust security, complex data handling, and scalability. Client-side APIs, on the other hand, are better suited for quick, user-facing tasks such as fetching static content or rendering visuals.
# Advantages of Server-Side APIs
- Enhanced Security: Sensitive logic, such as authentication, is handled on the server, reducing exposure to vulnerabilities.
- Centralized Updates: Changes to the API logic are made on the server, instantly affecting all connected clients.
- Scalability: Server-side APIs can scale with load balancers and distributed server clusters to handle high traffic volumes.
- Rich Functionality: Supports advanced operations, such as real-time analytics, data aggregation, and multi-database interactions.
# Advantages of Client-Side APIs
- Improved Speed: Eliminates the need for server requests by processing certain tasks locally, reducing latency.
- Offline Capability: With preloaded or cached data, client-side APIs can function even when there’s no network connection.
- Dynamic Interfaces: Enables faster rendering and updates for interactive UI components.
- Cost Savings: Reduces server load since some processing is handled on the client side.
# Challenges of Server-Side APIs
- Latency Issues: Requests to the server may introduce delays, especially for users with slow internet connections.
- Complex Deployment: Maintaining server-side APIs requires infrastructure, monitoring, and periodic updates.
- Scaling Costs: As traffic grows, scaling the backend infrastructure can become expensive.
# Challenges of Client-Side APIs
- Security Risks: Client-side APIs can expose sensitive information (e.g., API keys) to users or attackers.
- Device Dependence: Performance depends on the user’s device capabilities, which can lead to inconsistent experiences.
- Limited Functionality: Restricted to tasks that don’t require secure backend processing, such as database queries.
# When to Use Server-Side APIs?
Server-side APIs are ideal for applications that require:
- Data Security: For handling sensitive user data, such as payment processing, authentication, and financial transactions.
- Complex Business Logic: When operations require querying multiple databases, performing calculations, or generating reports.
- Cross-Platform Consistency: A centralized server-side API ensures that all client platforms (mobile, web, etc.) access the same data and functionality.
- Scalability Needs: For applications expected to handle a large number of concurrent users, server-side APIs are better suited.
# When to Use Client-Side APIs?
Client-side APIs are suitable for applications that prioritize:
- Real-Time Interactions: For rendering user interfaces or performing actions that require immediate feedback.
- Offline Functionality: Applications like media players, document editors, or games that need to work without a network connection.
- Quick Data Fetching: Fetching public data or static content directly, without involving a server.
- Cost Efficiency: Reducing the workload on servers by offloading non-sensitive tasks to the client.
# Combining Server-Side and Client-Side APIs
In most modern applications, a hybrid approach is used, combining the strengths of both server-side and client-side APIs. For instance:
- A server-side API handles secure authentication and provides encrypted user data.
- A client-side API renders user interfaces and updates the app’s state dynamically.
- The server-side API periodically syncs data with the client, ensuring accuracy and consistency.
Key Features of Server-Side APIs
For server-side APIs to function effectively, they should incorporate the following features:
# Authentication and Authorization
Secure access is vital for server-side APIs. Implementing protocols like OAuth2, JWT (JSON Web Tokens), or API keys ensures that only authorized users and systems can access sensitive data.
# RESTful Architecture
Most server-side APIs follow REST (Representational State Transfer) principles, making them stateless, resource-based, and easy to use. RESTful APIs are designed to work seamlessly with standard HTTP methods like GET, POST, PUT, and DELETE.
# Error Handling
A robust error-handling mechanism ensures that server-side APIs return meaningful error messages and HTTP status codes. This helps developers troubleshoot issues quickly.
# Rate Limiting
To prevent abuse or overload, server-side APIs implement rate limiting, which restricts the number of requests a client can make within a specified time frame.
# Versioning
API versioning ensures backward compatibility, allowing older versions to remain functional while newer ones introduce updated features and enhancements.
# Data Compression
Server-side APIs often compress data before sending it to the client to reduce bandwidth usage and improve response times.
Best Practices for Developing Server-Side APIs
When building server-side APIs, following best practices ensures reliability, security, and scalability:
# Design for Scalability
Plan for future growth by designing APIs that can handle increased traffic. This may involve implementing caching mechanisms, database optimization, and load balancing.
# Prioritize Security
Encrypt sensitive data, validate inputs and monitor API usage for suspicious activity. Regular security audits and penetration testing can identify vulnerabilities.
# Follow RESTful Standards
Adhering to REST principles ensures that APIs are easy to understand, use, and maintain. This also enhances compatibility with a wide range of clients.
# Optimize Performance
Use techniques like database indexing, query optimization, and caching to minimize response times. Monitor API performance regularly to identify bottlenecks.
# Document the API
Comprehensive documentation makes it easier for developers to understand and integrate the API. Use tools like Swagger or Postman to generate interactive API documentation.
How Shiv Technolabs Can Help?
At Shiv Technolabs, we specialize in crafting robust, scalable, and secure server-side APIs that power modern applications. Our expertise spans across industries, helping businesses harness the potential of server-side APIs to deliver exceptional user experiences.
# Why Choose Shiv Technolabs?
- Expertise in React Native Development: As leading React Native app development experts, we integrate server-side APIs with React Native frameworks to deliver seamless app performance.
- Custom API Development: We tailor API solutions to meet your business requirements, ensuring scalability and security.
- End-to-End Services: From design and development to deployment and maintenance, we provide comprehensive React Native app development services.
- Focus on Security: Our server-side APIs are built with robust authentication mechanisms to protect sensitive data and prevent unauthorized access.
Whether you’re building a new application or enhancing an existing one, Shiv Technolabs has the expertise to deliver server-side APIs that meet your goals.
Future of Server-Side APIs
The evolution of server-side APIs is set to continue with advancements in AI, machine learning, and cloud computing. Here are some trends shaping the future:
# GraphQL Adoption
GraphQL is gaining popularity as an alternative to REST, offering flexible and efficient querying capabilities that allow clients to request only the data they need.
# Serverless Architectures
Serverless technologies like AWS Lambda and Google Cloud Functions enable APIs to run without dedicated servers, reducing infrastructure costs and simplifying scaling.
# AI-Powered APIs
AI integration will enable APIs to perform more advanced tasks, such as predictive analytics, natural language processing, and image recognition, further enhancing app functionality.
# Improved Real-Time Capabilities
Technologies like WebSockets and SSE are making real-time data updates more efficient, paving the way for more interactive and dynamic applications.
To Sum Up
Server-side APIs are the backbone of modern app ecosystems, enabling efficient communication, secure data handling, and seamless cross-platform integration. By understanding their role and following best practices, businesses can build applications that deliver exceptional user experiences.
At Shiv Technolabs, we are committed to helping businesses leverage the power of server-side APIs through our expertise in React Native app development UAE and tailored API solutions. Whether you’re looking to enhance security, scalability, or performance, our team of React Native app development experts is here to help.
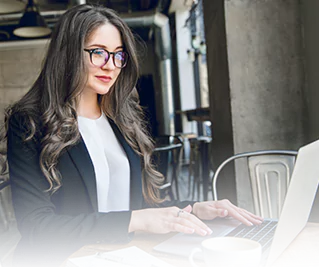