Table of Contents
In today’s digital landscape, real-time applications have become essential for delivering instant, engaging user experiences. Real-time updates—whether for chat, notifications, tracking, or data synchronization—are crucial across various app types. This guide dives into creating a real-time mobile app using React Native, covering the essential tools, libraries, and methodologies needed for an efficient implementation.
Why Build Real-Time Apps?
Real-time applications respond immediately to user inputs and external data updates, fostering interactivity and engagement. Apps in social media, e-commerce, gaming, and collaborative platforms benefit tremendously from real-time features, making it a compelling choice for any mobile app developer.
Prerequisites
Before diving into building real-time apps, it’s essential to have:
- Basic knowledge of React Native: Familiarity with its core components and hooks.
- Node.js and npm: Required for managing packages and dependencies.
- Backend services: While some methods allow peer-to-peer communication, most real-time apps rely on a backend for data storage and processing.
Step 1: Setting Up a Basic React Native Project
To get started, let’s set up a simple React Native environment.
1. Install React Native CLI:
npm install -g react-native-cli
2. Create a New React Native Project:
npx react-native init RealTimeApp
This forms the base for your app. Now, let’s dive into the tools you’ll need for real-time features.
Step 2: Choosing Real-Time Libraries and Technologies
Implementing real-time features in React Native typically involves libraries like WebSockets, Firebase, or SignalR.
1. WebSockets
WebSockets provide a protocol that enables two-way communication between a client and a server. This makes WebSockets suitable for real-time applications, as they allow the server to push updates directly to the client.
- Popular Libraries: react-native-websocket or socket.io-client
Implementation Example
import { useEffect, useState } from 'react'; import io from 'socket.io-client'; const socket = io("http://your-server.com"); function RealTimeComponent() { const [message, setMessage] = useState(""); useEffect(() => { socket.on("newMessage", (data) => { setMessage(data); }); return () => { socket.off("newMessage"); }; }, []); return <Text>{message}</Text>; }
2. Firebase
Firebase is an excellent choice for real-time applications due to its real-time database. It simplifies data synchronization and doesn’t require additional server setup.
Implementation Example:
import firebase from "firebase/app"; import "firebase/database"; // Initialize Firebase const firebaseConfig = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_AUTH_DOMAIN", databaseURL: "YOUR_DATABASE_URL", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_STORAGE_BUCKET", messagingSenderId: "YOUR_MESSAGING_SENDER_ID", appId: "YOUR_APP_ID" }; if (!firebase.apps.length) { firebase.initializeApp(firebaseConfig); } const RealTimeComponent = () => { const [data, setData] = useState(null); useEffect(() => { const db = firebase.database().ref("/data"); db.on("value", (snapshot) => { setData(snapshot.val()); }); return () => db.off(); }, []); return <Text>{data}</Text>; };
3. SignalR
SignalR by Microsoft is another excellent real-time library that’s especially effective for applications needing server push notifications, such as enterprise-level communication apps.
Step 3: Structuring the App for Real-Time Features
When designing a real-time app, consider organizing components to handle frequent updates. Using React hooks like useState and useEffect allows efficient state management in real-time applications.
- State Management: Use a state management library like Redux to handle frequent state changes, especially if your app has multiple real-time sources.
- Error Handling: Real-time applications require robust error handling. Implement retry mechanisms for failed requests and alerts for network disruptions.
- Optimizing Updates: To avoid overloading the app with updates, consider throttling or debouncing techniques for incoming real-time data streams.
Step 4: Implementing Real-Time Notifications
Notifications keep users engaged and informed, even when they’re not actively using the app.
Push Notifications with Firebase Cloud Messaging (FCM)
Firebase Cloud Messaging (FCM) enables reliable push notifications. Install @react-native-firebase/messaging for FCM support.
1. Setup FCM:
npm install @react-native-firebase/app npm install @react-native-firebase/messaging
2. Implement Notifications:
import messaging from '@react-native-firebase/messaging'; useEffect(() => { messaging().onMessage(async (remoteMessage) => { console.log("A new FCM message arrived!", JSON.stringify(remoteMessage)); }); }, []);
Step 5: Adding Real-Time Data Synchronization
Syncing data in real time across devices is vital for collaborative and multi-user apps.
- Conflict Resolution: Implement conflict resolution logic if multiple users attempt to update the same data.
- Offline Synchronization: Use Firebase or similar services to manage offline data, synchronizing once the device reconnects.
Step 6: Testing and Debugging Real-Time Features
Testing real-time apps can be challenging since issues may not always appear in a static environment.
- Network Simulation: Simulate poor network conditions using tools like Chrome DevTools or dedicated React Native testing tools.
- Monitoring and Logging: Real-time applications require constant monitoring. Use services like LogRocket, Sentry, or Firebase Analytics to track errors and performance issues.
Best Practices for Real-Time Apps with React Native
- Keep Data Updates Efficient: Only update necessary components to reduce processing time and power usage.
- Avoid Polling: While polling can simulate real-time behavior, it’s resource-intensive. Use WebSockets or Firebase for genuine real-time updates.
- Plan for Scalability: As your user base grows, so will the demands on your real-time backend. Choose a backend that supports scaling, like Firebase, AWS AppSync, or custom solutions on cloud providers.
Conclusion
Building real-time mobile applications with React Native can transform user engagement by providing immediate data feedback. By using tools like WebSockets, Firebase, or SignalR, you can deliver timely, dynamic features that adapt as users interact. With careful consideration of state management, notifications, and data synchronization, you’ll be well-prepared to launch a robust, real-time app that keeps users coming back.
For businesses looking to elevate their mobile app presence with dynamic, real-time capabilities, Shiv Technolabs is here to make it happen. As a leading React Native app development company in UAE, we bring expertise in building responsive, high-performance applications that meet the demands of today’s fast-paced digital landscape. Our React Native development services in UAE are designed to deliver scalable, engaging mobile experiences that keep users connected and informed in real time. Partner with Shiv Technolabs and transform your app vision into a seamless, real-time experience for your users.
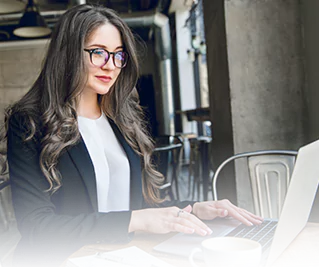