Table of Contents
WordPress powers over 40% of all websites, and with its reach comes the need for functionality that off-the-shelf plugins can’t always provide. That’s where custom plugins come in. If you’re looking to create a custom plugin in WordPress, this guide breaks it down from the first file to your first working feature.
By the end of this guide, you’ll understand how to develop a WordPress plugin from scratch, organize your code properly, and write your first custom feature. This post focuses on the first half of the process and is perfect for those working on creating a plugin in WordPress for beginners.
What Is a WordPress Plugin?
A WordPress plugin is a PHP-based set of code that extends the core functionality of a WordPress website. Plugins run independently from themes and do not alter core files. With custom plugins, you control the logic, structure, and output, making them perfect for unique business needs in WordPress development.
Plugins load via hooks—actions and filters—that tie into WordPress at predefined points. This flexibility allows developers to add or modify features without affecting the core.
Skills and Tools Needed to Create a Custom WordPress Plugin
To develop a WordPress plugin, you need a basic understanding of:
- PHP (object-oriented programming helps)
- WordPress functions and hook system
- HTML and CSS for layout (if UI is needed)
- JavaScript (for interactive features)
Recommended tools:
- Local development server (LocalWP, XAMPP, MAMP)
- Text editor (VS Code, Sublime Text)
- Access to a WordPress install for testing
Also Read: How Much Does It Cost to Build a WordPress Website?
Build a Custom WordPress Plugin in 2025: Step-by-Step Guide
Building a custom WordPress plugin means creating a standalone set of PHP files that plug into your site and handle specific functionality. You’re not just writing random code—you’re creating a self-contained tool that interacts with WordPress the right way.
This process lets you solve problems that off-the-shelf plugins can’t handle. Whether it’s integrating a third-party system, creating custom dashboards, or controlling a feature in a way that’s unique to your workflow, writing your plugin gives you full control.
Step 1: Set Up Your Plugin Folder and Main File
Go to your WordPress installation directory:
wp-content/plugins/
Create a new folder for your plugin. For this example:
my-custom-plugin
Inside that folder, create your main PHP file:
my-custom-plugin.php
Add the plugin header in this file:
<?php /* Plugin Name: My Custom Plugin Description: A basic custom plugin example. Version: 1.0 Author: Your Name */
This tells WordPress how to recognize and display your plugin in the dashboard.
Step 2: Activate the Plugin
Go to the WordPress admin panel → Plugins. You should now see My Custom Plugin listed.
Click Activate. Your plugin is now live, even though it doesn’t do anything yet.
Step 3: Add Your First Custom Function
Let’s write a simple function to confirm your plugin works. This first feature will print a message at the bottom of each page.
function my_custom_plugin_footer_message() { echo '<p style="text-align:center;">Thanks for visiting our site.</p>'; } add_action('wp_footer', 'my_custom_plugin_footer_message');
Now, load any frontend page. You’ll see the message appear in the footer. This shows that your plugin hooks into WordPress correctly and executes your code. This is your first step in WordPress plugin coding.
Step 4: Organize Your Plugin with a Proper Structure
As plugins grow, managing code in one file becomes messy. It’s better to create a separate folder called /includes
and place all functions there.
1. Inside your plugin folder, create /includes/
2. Move your logic into a new file: /includes/functions.php
3. Then, in my-custom-plugin.php
, include that file:
require_once plugin_dir_path(__FILE__) . 'includes/functions.php';
Now your plugin code stays clean, organized, and easier to expand. This is an important principle in WordPress custom plugin development.
Step 5: Creating an Admin Settings Page
Most plugins need some configuration. To support that, you’ll create a custom admin settings page. This page will allow users to control how the plugin behaves.
Add this code inside your functions.php
or create a new file like admin-settings.php
in the /includes
folder:
function my_custom_plugin_menu() { add_menu_page( 'My Plugin Settings', 'Custom Plugin', 'manage_options', 'my-custom-plugin-settings', 'my_custom_plugin_settings_page', 'dashicons-admin-generic', 80 ); } add_action('admin_menu', 'my_custom_plugin_menu'); function my_custom_plugin_settings_page() { ?> <div class="wrap"> <h1>Custom Plugin Settings</h1> <form method="post" action="options.php"> <?php settings_fields('my_plugin_options_group'); do_settings_sections('my-custom-plugin-settings'); submit_button(); ?> </form> </div> <?php }
This code registers a menu item called “Custom Plugin” and links to a simple settings form. You can expand this by registering fields using add_settings_field()
. This step allows your users to interact with plugin behavior through the admin panel.
Step 6: Secure Your Plugin
Security must be a priority when writing custom plugins. Even small features can open doors if not handled properly.
Follow these practices:
# Sanitize all input:
Use functions like sanitize_text_field()
, esc_attr()
, and esc_html()
before saving or displaying data.
# Escape output:
Always escape user data before rendering it in HTML to avoid XSS attacks.
# Use nonces in forms:
Protect admin forms from CSRF using wp_nonce_field()
and check_admin_referer().
Example of using a nonce in your form:
<?php wp_nonce_field('my_plugin_save_settings', 'my_plugin_nonce'); ?>
And when processing:
if (isset($_POST['my_plugin_nonce']) && wp_verify_nonce($_POST['my_plugin_nonce'], 'my_plugin_save_settings')) { // Safe to process }
Whether you create a custom plugin in WordPress for yourself or a client, skipping this step creates risk. Secure coding ensures long-term stability and trust.
Step 7: Add Translation Support
To support multiple languages, make your plugin translation-ready. Wrap all user-facing text in translation functions:
__('Text to translate', 'my-custom-plugin'); _e('Text to translate', 'my-custom-plugin');
In your main plugin file, add:
function my_custom_plugin_load_textdomain() { load_plugin_textdomain('my-custom-plugin', false, dirname(plugin_basename(__FILE__)) . '/languages'); } add_action('plugins_loaded', 'my_custom_plugin_load_textdomain');
Then generate a .pot
file using a tool like Poedit or WP-CLI. Save it inside /languages
. With this in place, you’ve supported localization the right way. This helps make your WordPress plugin from scratch usable worldwide.
Step 8: Load Scripts and Styles
If your plugin requires custom styles or JavaScript, load them properly using wp_enqueue_script()
and wp_enqueue_style()
. Example:
function my_custom_plugin_assets() { wp_enqueue_style('my-plugin-style', plugins_url('assets/style.css', __FILE__)); wp_enqueue_script('my-plugin-script', plugins_url('assets/script.js', __FILE__), array('jquery'), null, true); } add_action('wp_enqueue_scripts', 'my_custom_plugin_assets');
Use admin_enqueue_scripts
if you only want to load assets inside the WordPress admin. This keeps your WordPress plugin coding efficient and avoids loading assets where they aren’t needed.
Step 9: Testing and Debugging
Once your plugin has several features, test everything before going live. Enable debugging in your wp-config.php
file:
define('WP_DEBUG', true); define('WP_DEBUG_LOG', true);
Log errors to wp-content/debug.log
and watch for notices or deprecated functions.
Use tools like:
- Query Monitor – for tracking database queries and hooks
- Developer plugin – helps check for best practices
- WP-CLI – for command-line-based plugin checks
Thorough testing is essential to build a WordPress plugin from scratch that behaves consistently across environments.
Step 10: Prepare for Compatibility
To keep your plugin future-safe:
- Follow WordPress coding standards
- Prefix all functions and variables to avoid naming conflicts
- Test with the latest WordPress versions
- Keep documentation up to date
A plugin that follows good structure and naming will last longer and be easier to maintain.
Step 11: Package and Share Your Plugin
Once your plugin works and passes all tests, package it for others to use. Zip your plugin folder and share it via email, GitHub, or manually upload it through the WordPress admin.
If you want to share it via WordPress.org:
- Add a
readme.txt
file - Make sure your plugin uses a GPL-compatible license
- Follow the guidelines provided by the WordPress Plugin Developer Handbook
This step-by-step guide shows how to build a custom WordPress plugin from scratch using the correct methods and coding standards. Whether you’re adding features to your own site or building tools for clients, this foundation will help you create clean, reliable plugins every time.
Build a Custom WordPress Plugin with Shiv Technolabs
At Shiv Technolabs, a trusted WordPress development services provider, we specialize in creating WordPress plugins tailored to unique business needs. A custom WordPress plugin isn’t just about adding features—it’s about delivering reliable, high-performing solutions that integrate seamlessly with your website.
When you work with our team, we don’t simply modify existing plugins or provide one-size-fits-all tools. Instead, we start by understanding your goals. Whether you need a new form feature, a custom dashboard integration, or enhanced eCommerce functionality, we build plugins from the ground up.
Here’s how we typically approach custom WordPress plugin development:
1. Discovery and Planning: We begin by identifying your exact requirements, evaluating existing solutions, and planning the plugin’s architecture.
2. Clean, Modular Code: Our WordPress developers follow coding standards, ensuring that every function is well-structured and that the plugin is easy to maintain.
3. Focus on Performance and Security: Each plugin is crafted to run efficiently without bloating your site. We implement rigorous security measures, from input sanitization to output escaping.
4. User-Friendly Admin Interfaces: If your plugin requires settings or configuration, we create intuitive admin pages that allow you to manage options with ease.
5. Testing and Ongoing Support: After development, we thoroughly test the plugin to ensure it works seamlessly on your site. Plus, we offer ongoing maintenance and updates as needed.
By choosing Shiv Technolabs, you gain a reliable partner who ensures your custom plugin not only meets your immediate needs but also remains stable, secure, and scalable as your business grows.
Also Read: 10 Reasons to Outsource WordPress Development to a White Label Partner
Conclusion
You’ve now completed every step required to develop a WordPress plugin from concept to execution. You started with folder setup, added functional code, created admin settings, secured the logic, loaded assets properly, and prepared the plugin for real-world use.
Whether you’re building tools for your site or releasing a plugin for wider use, this foundation helps you build smarter and more efficiently.
If you’re looking for expert support to create a custom plugin in WordPress or need help scaling an existing one, Shiv Technolabs is ready to support your goals. Our team builds reliable, well-structured plugins tailored to your exact needs, following clean development practices from start to finish. Reach out to us now!
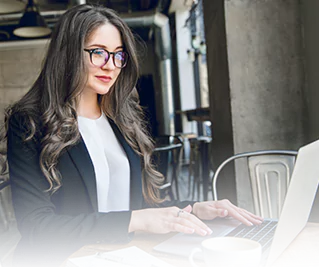